How to Disconnect and Remove a Client's Stripe Account
We've managed to create the functionality to have a logged-in user in our app connect with Stripe. In this lesson, we'll look to visually indicate that the user is in the connected state and have an action responsible for allowing the user to disconnect from Stripe.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
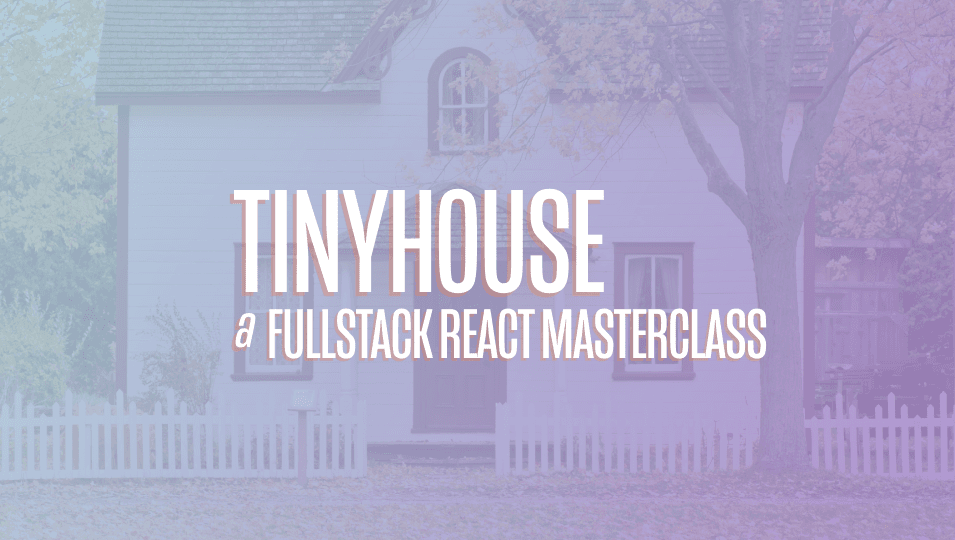
[00:00 - 01:07] we've managed to create the functionality to have a logged in user in our app connect with Stripe. In this lesson, we'll look to visually indicate that the user is in the connected state and have an action also responsible in disconnecting the user from Stripe. At this very moment, when we query for the user object in our user page, we're already querying for the has wallet field within the user GraphQL object. This has wallet field simply indicates the presence of the user's wallet ID field in the document in the database. If it exists, it means we have the Stripe user ID of the user. In the user page, or in the user component page, if I place a console log for the user object being queried, at this moment in time for my user profile, the has wallet field returns true. And that's because I've connected with Stripe in the previous lesson. We should have UI in the user profile section that reflects the user is in the connected state with Stri pe.
[01:08 - 01:57] If we take a look at our user profile components, we can see that we only show the additional details section when the user is logged in, which is what we want. However, the information we show, such as the button to connect to the information telling the user to become a host, should only be shown when the user hasn't yet connected with Stripe. So we'll take the upper paragraph within the section, the connect button, and the secondary paragraph and place it within a constant element above, we'll call just additional details, and we'll wrap it all within a fragment. We'll say if the has wallets field in the user object available as props is true, we'll simply render an empty fragment. If not, we'll render the contents we had before.
[01:58 - 03:07] And we'll place the additional details element within the additional details section element. If we take a look at the user page right now, in the user profile section, we 'll see nothing after the additional details title. This is because we're in the connected state or this user at this moment has wallets. Okay, so let's now look to show information to the user that they have connected with Stripe. So in the user profile components, we'll first import one other component from ant design that we'll use called the tag component. We'll also import the utility function format listing price that we've used before to help format price number values, and we'll import it from the libutels file. In our additional details constant element, we'll say when the user has wallets, we'll display a green tag within a paragraph that says Stripe registered.
[03:08 - 03:33] We'll have another paragraph dedicated to the income of the user with which we 'll be able to access from the income field in the user object. We'll do a small conditional and say if this user income exists, we'll use the format listing price function and place the income value within. Otherwise, we'll just simply say zero dollars.
[03:34 - 03:58] We'll have a button responsible in disconnecting from Stripe. And we'll have a secondary paragraph that will tell the user that if you disconnect from Stripe, this will prevent other users from booking listings that you might have already created.
[03:59 - 04:30] We'll talk more about this when you build a functionality for bookings, but it'll be an important message to tell the user. Now note that we're using the format listing price function to help take the income within the user object in sense and show it in dollar formats within a nicer particular format.
[04:31 - 04:50] Now this is outside the context of listing, so we could perhaps rename this function, but we'll just leave it as is. If we take a look at our user page now, we'll see the new section be shown since this user is in the connected Stripe state. Great. Now let's focus on providing the capability to disconnect from Stripe.
[04:51 - 05:47] We have the disconnect Stripe mutation set up on the server, so let's first create the mutation document in our client. We can replicate the connect Stripe mutation document we've created in the last lesson, change the name of the mutation and the constant, and we'll remove the need to pass in an argument since the disconnect Stripe mutation doesn't expect an input. With this mutation, we'll also want the has wallet field from the viewer object in the API to be returned. And in the lib graph ql mutations index file, we'll re-export the disconnect Stripe mutation document. We'll head over to the terminal to auto generate our types. We probably won't have to regenerate our schema since we did so in the last client lesson, but we'll go ahead and run the code gen schema command again just in case.
[05:48 - 06:31] And when complete, we'll run the code gen generate command to auto generate the TypeScript definitions. We'll use this disconnect Stripe mutation in the components that we expected to be used, which is the user profile component. In user profile, we'll import the use mutation hook from react Apollo. We'll import the disconnect Stripe mutation document.
[06:32 - 06:53] And the relevant TypeScript definitions for Adapt mutation. Since there are no variables for the disconnect Stripe mutation, we'll only import the data interface.
[06:54 - 08:19] In the beginning of our user profile components function, we'll use the use mutation hook, pass along the appropriate type definitions, and destruct the disconnect Stripe mutation function. The only result field we 'll use will be the loading state of our mutation. To handle the success in error states of the mutation, we'll look to either display a success notification or an error message. Since we're not going to render anything for either of these conditions, we'll simply handle this by using the on completed and on error callback functions as part of the mutation results. We'll import the display success notification and the display error message functions from the libutils file to help us here. In the on completed callback function, we'll check for the data object and if the disconnect Stripe object within data exists, and if so, we'll simply run the display success notification message and we'll say you've successfully disconnected from Stripe.
[08:20 - 08:43] For the description, we'll say you'll have to reconnect with Stripe to continue to create listings. And in the on error callback, we'll use the display error message utility function and say something like sorry, we weren't able to disconnect you from Stripe.
[08:44 - 09:24] Please try again later. Similar to how in our connect Stripe mutation, we want to ensure the viewer state object available in our client is updated when the user has successfully disconnected from Stripe. So we'll do something similar here and we'll have the viewer and set viewer object as available as props in this component. And the type of the viewer instance will be the viewer interface will import from the lib types file.
[09:25 - 10:26] In our mutation on completed callback, we'll use the set viewer function, apply the spread operator to pass in the values of the viewer object and update the has wallet property with that within from data dot disconnect Stripe. Now let's make sure the viewer and set viewer props are going to be passed down to this user profile component. So in the parent app component, we'll make sure to pass the set viewer function available here down to the user page components . In the user components, we'll declare the set viewer prop that is going to be passed in.
[10:27 - 11:13] And we'll pass the viewer and set viewer props further down to the user profile components. And in the user profile components, we'll now look to use the disconnect Stripe mutation function and the loading result from the mutation. We'll place the loading result as the value of the loading prop in our disconnect Stripe button. And the on click handler of the button will trigger a callback that calls the disconnect Stripe mutation function.
[11:14 - 11:49] And that should mostly be it. Let's see what happens now. In our user profile section, when I click the disconnect Stripe button, we'll see the success notification telling us that the mutation was successful. And the network logs tell us that the request was made with status code 200. However, in our user page, the UI remains the same. But when we refresh the page, we'll get the appropriate section that shows we're not connected with Stripe any longer. Why is this happening?
[11:50 - 13:18] This is because after our mutation was successful, we haven't re queried the user query in the parent component, which is why the UI remains the same. So in the parent user component, we'll destruct the refetch property from the use query hook. And we'll set up an async function in that component called handle user refetch that will simply trigger the refetch function. We'll pass the handle user refetch function as a prop of the same name to the user profile components. In our user profile component, we'll state that the handle user refetch function is to be expected as a prop. And in our on completed callback of the disconnect Stripe mutation, we 'll trigger the handle user refetch function at the end. So now let's see how this would work. First, we'll go through the connection process to have our user profile reconnected with Stripe. And upon disconnecting, right after the mutation is successful, the user query will be refetched to get the most up to date information of the user, hence having our UI also being the most up to date state.
[13:19 - 13:27] Great. And that's pretty much it. For a user that's now logged in, we have the capability to connect and disconnect from Stripe.