Module 9 Summary
This lesson is a summary of the work we've done in Module 9.0.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL - Part Two with a single-time purchase.
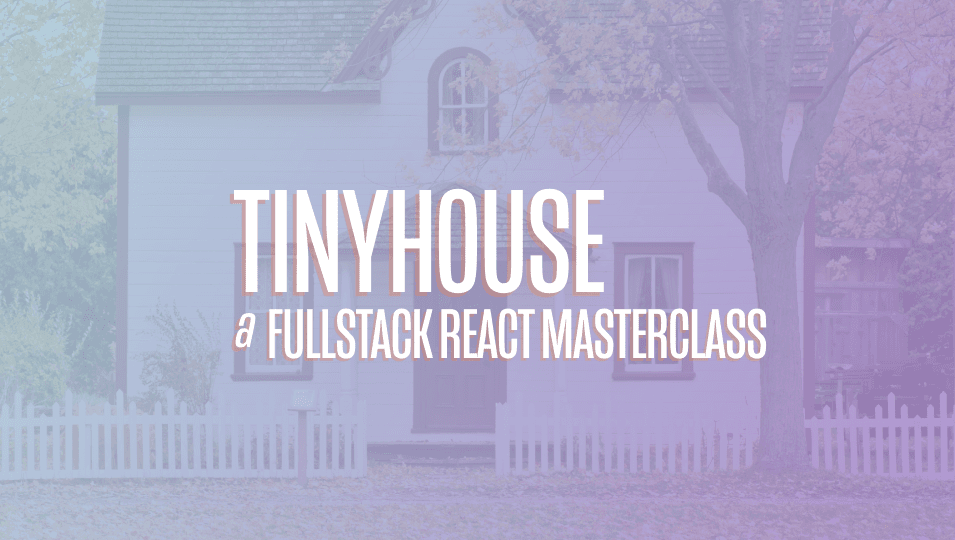
[00:00 - 00:18] In this module, we built in what we've done in the previous module, and now we 'll allow the user to basically query for listings that pertain to certain locations. We've updated the type definitions for the listings root level query field, and we've simply added an optional argument called location.
[00:19 - 00:38] And when provided, our listings graph QL field would essentially return the listings that pertain to the location that was searched for. If we take a look at our listing resolvers map, and in the actual listings graph QL query, everything below remains the same.
[00:39 - 00:51] However, in the very beginning, we check to see if this location argument exists. And if it does, we attempt to determine what the country, administration area, and city for this location is by using Google's geocoding API.
[00:52 - 01:09] And once all of this is determined, we then aim to build this query object that we use to actually find the listings in our listings collection that pertain to this certain query. In other words, find the listings in which the city, admin, and country is what the user is looking for.
[01:10 - 01:45] In the Google object we've set up in our lib API folder, the new function we've established is called geocode, and essentially runs the geocoding API available from Google Map Services, and we pass in the location, or in other words, the address that the user is searching for. And once this response exists, we parse the response given to us from Google's geocoding API, and we determined to find the country, the administration area level one, as well as the city, which is either the locality or the postal town from the information desk return.
[01:46 - 02:13] And now when we move over to our client application, the main change that we've made here is essentially to build out the listings component, which is the component that gets shown when the user attempts to access the listings route and provides a valid location URL parameter. And it's in this component that we essentially retrieve this location URL parameter and run the listings query to find the listings that pertain to this location.
[02:14 - 02:27] When this page is first loaded, we automatically set the filter to be from low to high pricing, and the page to be one. When this query is loading, we show the skeleton that we've established a custom skeleton page just for this component.
[02:28 - 02:40] And when it errors, we show an error banner up top telling the user to something went wrong. And when data is available, we essentially show the list of listing cards where every listing card is basically a summary of what the listing is.
[02:41 - 03:02] In addition, we do show two separate sections at the top, which is the pag ination section, which can give the user the capability to navigate from page to page and the filter section, which gives the user the capability to change the filter that's available. If for some reason or another that the query is successful and no listings exist, it probably means that no listings have been created.
[03:03 - 03:09] So we tell the user that as well. And lastly, one last thing we did at the end of this module was particularly important.
[03:10 - 03:26] And in our actual MongoDB Atlas dashboard, we've provided an index to support the effect of the efficient execution of queries from the listing collection. And we define an index for the three fields we want queried, the country, admin and city fields.