Learn to build performance-critical Rust apps
The Rust language is a way to write incredibly fast - and safe - code. It's being used to build tools at Google, Facebook, Amazon, and many other companies where performance is critical.
While there are some good resources on how to learn the Rust programming language by itself, what these other books don't teach is how to build applications with Rust.
Fullstack Rust solves that. In this book we show you how to use Rust to build incredibly fast web-servers, build command-line tools, and compile apps to run in the browser with Web Assembly (WASM).
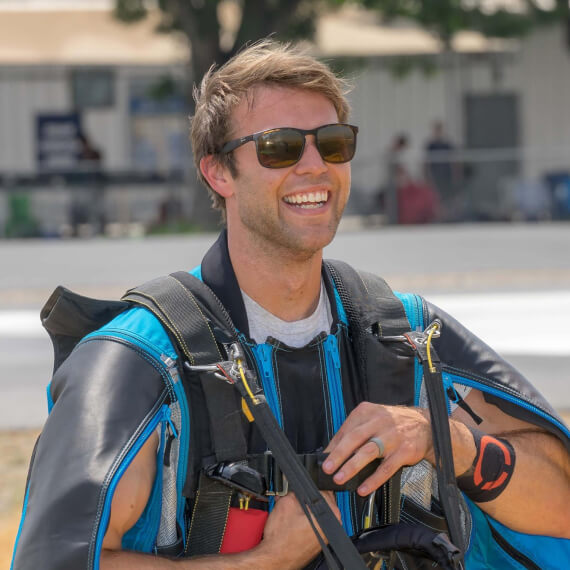
Meet the Author: Andy Weiss, Software Engineer at Google
I started my career as a Data Scientist and Software Engineer at Facebook before becoming the first engineer at Flexport.
I began working with Rust as a hobby before putting it into production while at Rollbar. I'm now working on Fuchsia at Google. In my work, I try to mix the academic rigor from a PhD at Princeton with pragmatism learned from shipping products at companies big and small.
In Fullstack Rust I've put together a book that will show you how to use the Rust ecosystem to build fast, secure, apps and tools.
Learn the techniques and tools to build realistic Rust applications
Rust has features that make it a fantastic tool for a number of tasks. Some highlights include:
- Performance
- Strong, static, expressive type system
- Fearless concurrency
- Great error messages
- Modern generics
- Memory safety
- Cross-platform
- C interoperability
- Compiles to WASM (WebAssembly)
Rust has a great set of documentation around the standard library. However, this book has a different focus - instead of trying to teach you just the Rust language, our goal is to build realistic applications and explore some of the techniques and tools available in Rust for accomplishing those tasks.
In the process of working through some common scenarios, you will also be able to learn Rust.
In the book, we teach with a gradual ramp-up from very simple to more complex programs as we build up our Rust toolbelt. Along the way, we'll show places where many people stumble and support you in finding your own way over these hurdles.
By the time you're done with this book, you'll be empowered to branch out and build your own Rust programs.
Mathew Varughese
Software Engineer at Stripe
As someone that is interested in systems programming, but did not know where to start, Fullstack Rust was a great way for me to get started.
The book is structured in a digestible way that teaches the what, how, and why of the Rust programming language.
Table of Contents
- Your First Rust App1
- Getting Started With Rust
- Making Our First Crate
- Testing Our Code
- Making A Web App With Actix2
- Rust Web Ecosystem
- Handling Requests
- Understanding Rust Closures
- Adding State To Our App3
- Effectively Working with Locks
- Custom Error Handling
- Handling Path Variables
- ORMs with Diesel4
- The Diesel CLI
- Migrations
- How to Organize Your Code
- Web Assembly (Wasm) and Rust5
- Rust in the Browser
- The Smallest Wasm Library
- The Real Way to Write Wasm
- Command-Line Rust Applications6
- Building an MVP
- Adding Configuration Files
- Adding Sessions
- Rust Macros7
- Declarative Macros
- Procedural Macros
- Writing a Custom Derive
Purchase the book today
- PDF, EPub, and Mobi
- Completed code
- Access to our community
- Learn to build production apps
- Access to every book
- All code projects
- Access to our community
Samuel Wasswa
Technical Director at Bitways Limited
Rust is quite unique and Fullstack Rust does a great job of introducing the concepts that make the language excel without overwhelming the reader.
If I was to summarize the book in three words, they would be Practical, Concise and Precise. It's a great way to dive into the world of Rust.
I'm wondering...
What happens after I buy the book?
You'll be able to download the book and source code after checkout. You'll also receive an email from Gumroad giving you instructions on how to download it at any time.
How long is the book?
The book has 7 chapters totaling about 310+ pages.
Is the book complete?
Yes. The book is completely finished.
Are there free updates?
Yes! Buying now entitles you to free updates for at least one year after purchase
How do I download the book and updates?
If you've purchased the book, you can download it from your Gumroad library.
How up to date is the book?
The book is up to date with the latest version of Rust
What format is the book?
The book is in PDF, epub, and mobi format. It also comes with a large folder of example code
What if I don't like it?
If you're unhappy with the book for any reason, just reach out to us and we'll give you a full refund. There's no risk.