Using Specification Methods in Controllers
In this lesson, we're going to implement our Specification methods inside Controllers
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
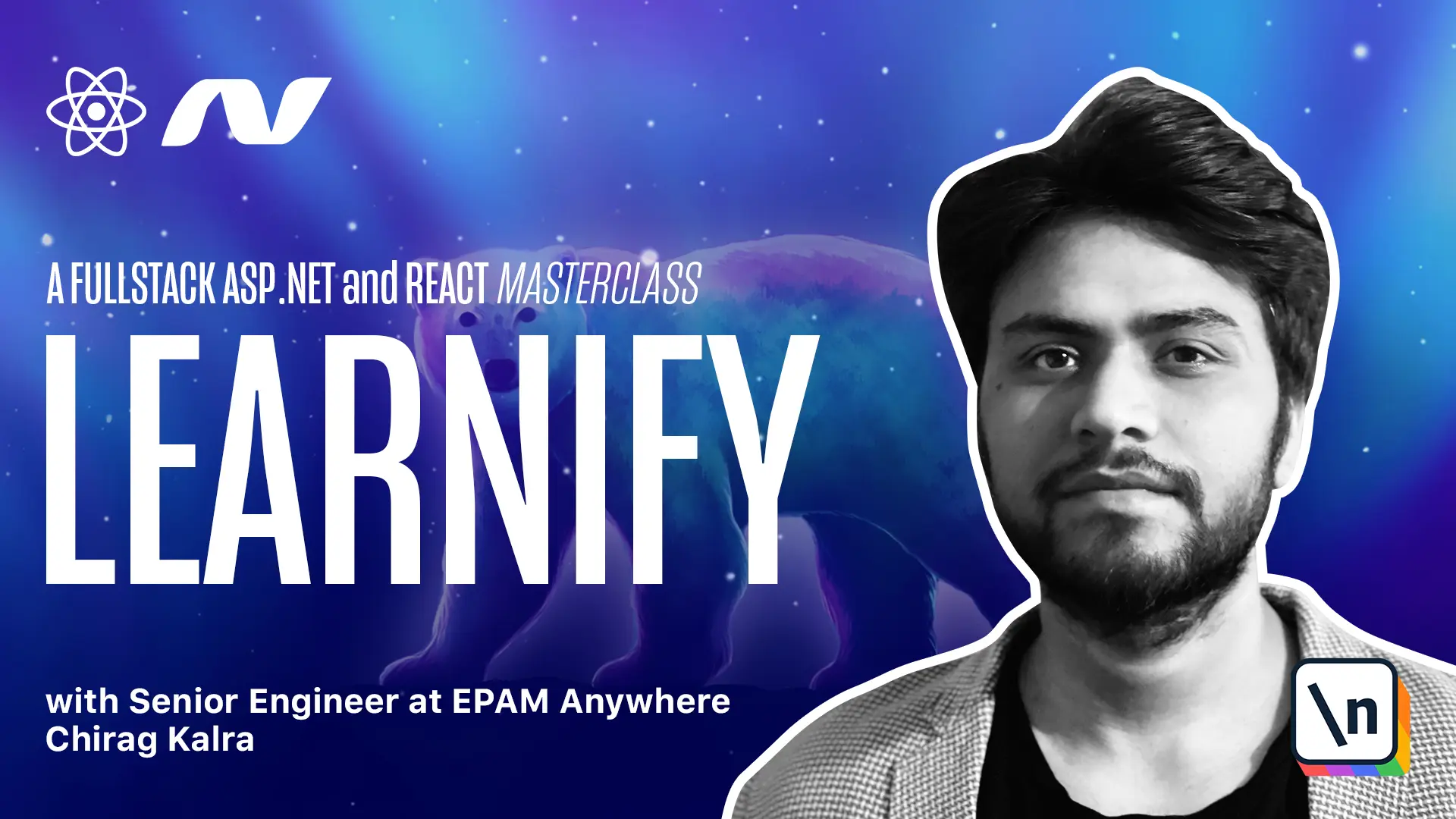
[00:00 - 00:16] We have created our specification interface, we have created the methods, now we simply have to inject it to our controllers. If we go to our courses controller, we are simply returning the list and a single entity without any navigation properties.
[00:17 - 00:32] By just looking at the name list all async, we don't know what kind of a list we are getting or which navigation properties it will have. Getting specifications gives us an opportunity to create some meaningful specification which we can pass to our generic methods.
[00:33 - 00:48] For example, I want to retrieve the categories along with the courses. So what I can do is inside our entity project, inside specifications, I can create a new specification with name, courses with categories specification.
[00:49 - 01:07] So let me create a new class and I will name it courses with categories specification. You can make it shorter as well but the whole point is to make things easier to understand.
[01:08 - 01:25] Our courses with category specification will derive from the base specification . And this time we will not pass dynamic type t because we know we want our courses so I will simply call it course.
[01:26 - 01:41] We see an error because inside our base specification, we do not have an empty constructor. We have one with the criteria but not an empty one and since we are not using any constructor here, we are getting an error.
[01:42 - 01:54] What I will do is create an empty constructor as well, generate constructor base specification. And now we see the error is gone.
[01:55 - 02:02] I will create an empty constructor here as well. So generate constructor, this empty one.
[02:03 - 02:14] Since we are deriving from the base specification, we have the access to this include method. And if you remember our include method wants an expression which returns an object.
[02:15 - 02:32] Since we have used the type course here, so if you use the include method, we will have access to all the navigation properties of our course. For example, we have category, we have learnings and requirements as well.
[02:33 - 02:40] So I will click on category. Now we can simply use this specification inside our controller.
[02:41 - 02:55] Let's go back to our courses controller and inside the get courses method, we need a new variable which will store our specification. So what I'll do is I will create a new variable called spec and it's going to be equal to new.
[02:56 - 03:22] So we have to create a new instance of it and I will call it courses with categories specification. And we can simply let's make sure the spellings are correct courses with category specification.
[03:23 - 03:37] Now we can import it using entity dot specifications. Now what we can do is we can pass this specification to our list all async method.
[03:38 - 03:45] We will see an error because this method does not accept any specs. For this we have created list with spec.
[03:46 - 04:00] So let's use that instead list with spec and we see the error is gone. Now we can use the same specification for our get course method as well.
[04:01 - 04:12] In get course method, we will have to use an expression which will check the ID . Let's go to our specification and this time generate a constructor which takes a criteria.
[04:13 - 04:30] Okay, so I will use this and select the constructor which takes in criteria. Here we can pass our parameter which in this case is ID of type GWT and inside our base we will pass the expression.
[04:31 - 04:40] Our expression is going to check the ID. So what we can do is X dot ID is equal to the ID that we have passed.
[04:41 - 04:50] Now if we go to our base specification, we have two constructors. One of them is the empty one which we just created and the second one takes a criteria.
[04:51 - 05:03] This one we can use when we only want to use the include methods and we do not want to use any expression and the second one takes a criteria. The criteria takes an expression as well.
[05:04 - 05:16] For example, the expression which we provided right now like this one and we can also pass the include statement in here because we have access to it. So we would like to include the requirements.
[05:17 - 05:36] So I will use include method. The first one will be C dot requirements and the second one will be learnings.
[05:37 - 05:48] Now if we go back to our courses controller, we can use the same spec. So let's copy it and paste it here.
[05:49 - 06:11] But this time we will provide the ID because our second constructor takes ID and it then works as a criteria. So basically when we are not providing any parameter, we are using this empty constructor, this one and when we are passing any parameter, we will use this constructor.
[06:12 - 06:21] So get by ID async does not accept any parameter. So we will change it to get entity with spec and we will pass this specification.
[06:22 - 06:46] Now let's create another specification for categories controller. So again, I will go to entity project and then go to specifications and this class will be called categories with courses specification.
[06:47 - 07:03] First of all, let's derive from base specification and this time it is for category. So I will call it category and this time we don't want any empty constructor because our get categories method does not require any navigation properties.
[07:04 - 07:13] So if I go to the categories controller and the get categories method, we do not want any navigation properties. So we do not have to use the empty constructor.
[07:14 - 07:31] So what we can do is we can create a constructor with a criteria and that is for our get category method. So we place this with ID and ID is of type int and the criteria is going to be the same.
[07:32 - 07:52] So what I'll do is X, which goes to X dot ID is equal to the ID that we are passing here. Inside here, we will again use the include method and this time it will add the courses navigation property.
[07:53 - 07:59] That's it. What I'll do is copy this name because writing this big name is going to be a task in itself.
[08:00 - 08:34] Let's go back to our categories controller and here let me create a new spec and this time it will be new categories with courses specifications and this one takes an ID because we do not have an empty constructor that's imported using entity.specifications and replace this method with get entity with spec and pass spec to it. Make sure the server is running.
[08:35 - 08:49] So let's go to the API project and dotnet watch run. In postman and let's run all the requests one by one.
[08:50 - 09:11] So let's start with get courses and we see that we have category and since we haven't added requirements and learning so we don't see it. So we see all the courses with the category because we have used the include method to include this category name navigation property.
[09:12 - 09:39] Let's try the get course request now and what I'll do is I will call it once again and copy this ID paste it here and again this time we see the requirements and learnings . We don't see category because we haven't included the navigation property for category.
[09:40 - 09:51] Now let's try the categories. This is a simple one since we don't want any navigation properties and yes we see ID and name everything's working fine and get category.
[09:52 - 10:04] Let's call the one category and see we see all the courses now. So after all this hard work we are exactly as the same place as we were in the last section.
[10:05 - 10:22] But now you know how to implement generic repository and if you want to create a larger project with multiple repositories using generic repository saves a lot of time and effort. If there is something confusing you right now don't worry in the next lecture we will go through the entire process.
[10:23 - 10:24] So I will see you in the next one.