Adding Code to Get Data from Category Table
In this lesson, we're going to add code to get data from Categories table
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
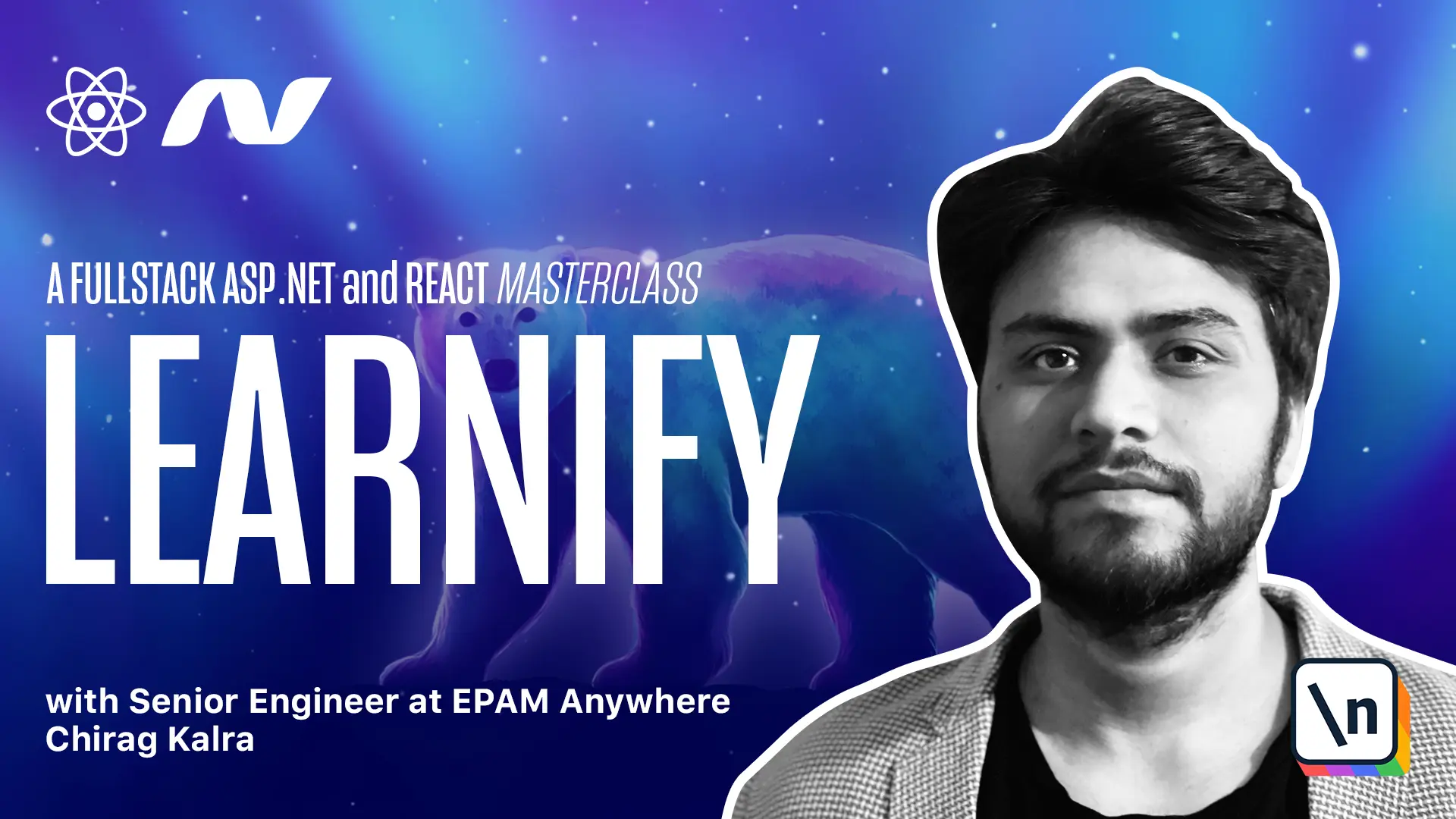
[00:00 - 00:08] We have seeded the data in our database in the last lecture. In this lecture we will see how to fetch data from our category stable.
[00:09 - 00:18] Unlike learnings and requirements, we want to call categories separately. Also, we want to see courses that belong to the same category.
[00:19 - 00:25] For that, we'll have to create a different controller. First of all, let's create a new interface inside.
[00:26 - 00:39] Interface is folder which is inside the entity project. New interface and let's call it i category repository.
[00:40 - 01:08] Inside this interface, first method will return list of categories. So, we can write task of ireadonlylist, readonlylist of type category and this will be called getCategories asin.
[01:09 - 01:18] Now, we'll have to import. Let's import it using system.threading.tasks.
[01:19 - 01:26] Let's import ireadonlylist from system.collections.generic. The second method will return a single category.
[01:27 - 01:51] So, let's make it task of category and we can name it getCategories by id using and it will receive an id which will be of type int. Now, inside the infrastructure project, we'll have to implement this interface.
[01:52 - 02:05] So, inside we can create a new c# class. Let's call it category repository.
[02:06 - 02:19] And this will derive from i category repository. Let's import it using entity.interfaces.
[02:20 - 02:32] We need to generate a new constructor. Let's create one constructor and use our store context here which will be called context.
[02:33 - 02:42] After this, we will initialize field from parameter here. Now, let's implement this interface using quick fix.
[02:43 - 02:53] Let's write our first method. We will use async in front of it because that is going to be an asynchronous method and remove this default line.
[02:54 - 03:09] And instead of this, we will return await. Just go context.categories.to list async.
[03:10 - 03:21] Let's import it using Microsoft. entity framework. Inside the second method, we will write async again and remove this line.
[03:22 - 03:37] Return await_context.categories.find async and we will pass the id that we are getting here. And this is simply it for the repository.
[03:38 - 03:56] We can now make our categories controller. Inside our API project, inside the controllers, let's create a new one and this one will be called categories controller.
[03:57 - 04:05] This will derive from our base controller. And let's generate a new constructor.
[04:06 - 04:18] This constructor will have our iCATICRE repository. So let's write iCATICRE repository and let's simply call it repository.
[04:19 - 04:27] We need to import it using entity.interfaces. And now initialize field from parameter.
[04:28 - 05:07] Let's write our get methods now on top. We will write HTTP get and below that, we will write public async task, which will be action result of i redone list, which will be of type categue. And let's call it get categue.
[05:08 - 05:17] We can implement it here before that. Let's import it using system.threading.tasks.
[05:18 - 05:30] And let's import action result from Microsoft.asp netcode.ndc. We need to import i redone list from system collections.generic.
[05:31 - 05:41] And finally, we need to import categue using entity. Let's make a new variable called categories, which will store the data for us.
[05:42 - 05:56] We can write await and let's go repository.getcategue's async. That's the method that we created inside our repository.
[05:57 - 06:06] And finally, we will return it wrapped inside our okay response. Let's return it.
[06:07 - 06:22] Now, let's make our second method. Our second method will accept an ID. So what we have to do is HTTP get with brackets and inside brackets, we need quotation marks.
[06:23 - 06:44] And finally, the curly brackets with ID. Let's write the method now. public, async, again task, action result, action result of type category.
[06:45 - 06:55] Now, let's call it get category. And this will accept an ID, which will be of type integer.
[06:56 - 07:10] We need a variable category, which will store our response. So let's make a variable category and it will await repository.getcoursesbyid async.
[07:11 - 07:14] And we will pass the ID. Sorry, not in capitals.
[07:15 - 07:27] And finally, we will return the category. Finally, we have to go to our startup class and add this repository into the services.
[07:28 - 07:44] So let's call services dot add scoped. This one is I category repository and the implementation is called category repository.
[07:45 - 07:55] And that's it. Let's open the terminal.
[07:56 - 08:01] Make sure you are inside the API project. And let's run.net.
[08:02 - 08:03] Watch. Run.
[08:04 - 08:12] Open postman and inside module three, open this get categories request and press send. Here we see all the categories, but we don't see the causes.
[08:13 - 08:35] Also, if we use the get category method and call the category with ID one, you see, we see this category, but we don't see the causes. Also, if we call this API courses request, which is in module one, we see all the properties, but we don't see learnings, category and requirements.
[08:36 - 08:40] How do we include properties from a different table? Let's take a look in the next lecture.