Technologies Used in this Course
In this lesson, we'll go through all the technologies we're using in this course
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
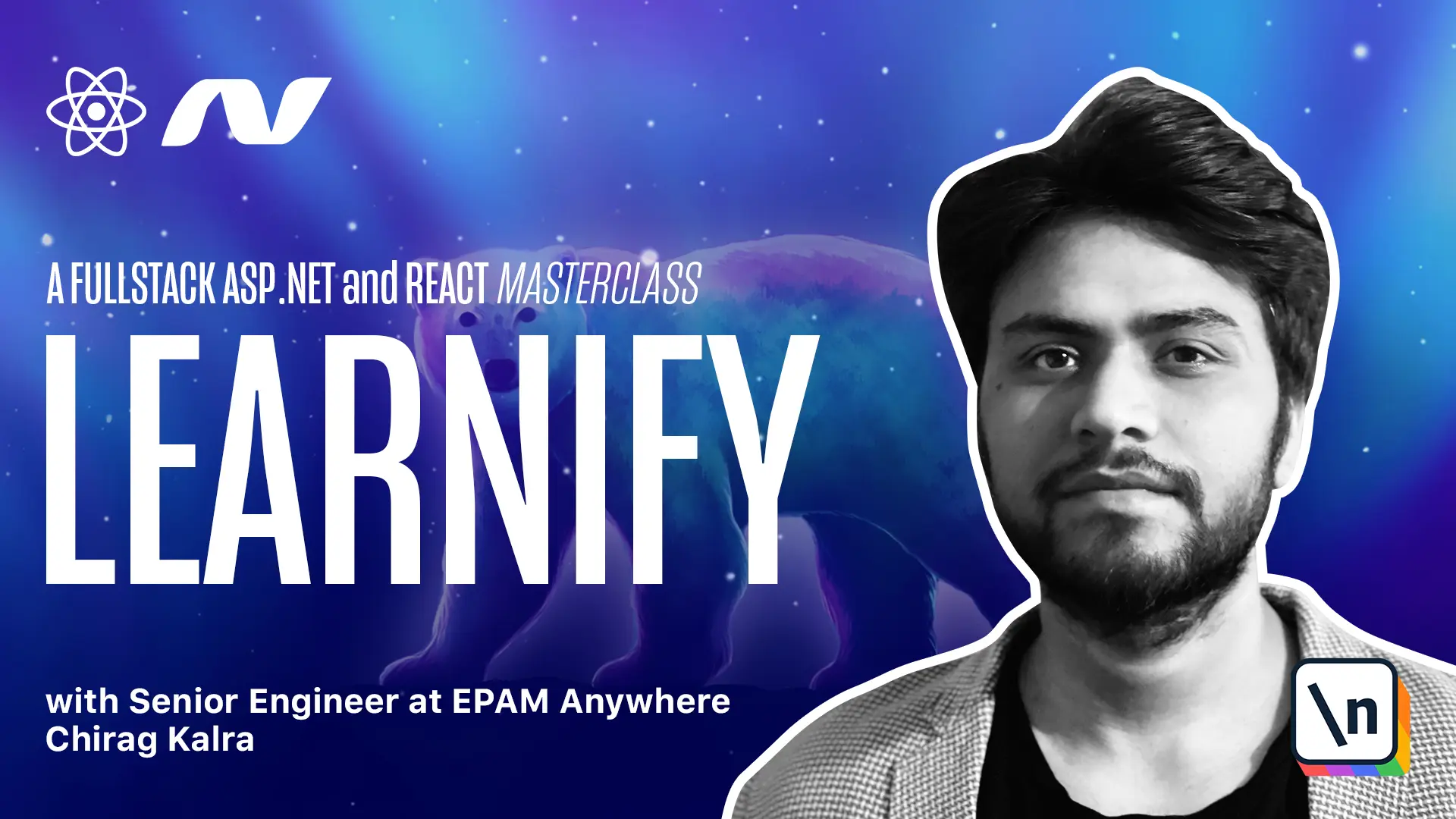
[00:00 - 01:06] Let's talk about technologies we are going to use in this course. In the front end, we will be using ReactJS, which is the most popular JavaScript framework. React is a good choice because it has a faster learning curve if you compare it to other libraries, such as Angular. React is backed by Facebook, which ensures that it's not going anywhere. In this course, we will be using React with TypeScript, which gives us type safety in compile time. It's amazing because it simplifies the JavaScript code and makes it easier to read and debug. It supports static typing, which means a variable has a defined data type and if you try to change it, it gives you an error. It's essential because as a developer, we want our code to be organized. It also supports all the latest JavaScript features, so there are no obstructions if you want to take advantage of all the new JavaScript features. Now let's talk about SAS. It stands for synt actically awesome style sheets. If you haven't heard of it, it is the more advanced version of CSS.
[01:07 - 01:51] It is a preprocessor scripting language that is interpreted or compiled into CSS. In short, it provides us more features than CSS and it's better for development . You can use variables, operators, placeholders and so much more using SAS. Now for the state management, we will be using Redux toolkit. It gives us all the features of Redux without the boilerplate code Redux has. In Redux toolkit, we have slices. So one component will have one slice, which will be responsible for everything. Actions, reducers and Thunk. They used to exist in different files before, but now all the related code will be centralized inside Redux toolkit.
[01:52 - 02:29] Now this is it what we are going to use in Declined side. Now let's talk about our backend. ASP.NET is going to be our protagonist. It has gained so much popularity that it became the most popular backend framework in 2020. It is way faster than any other backend service. Also, it's considered perfect technology for enterprise softwares. Now you can imagine how much highly rated this framework is. This is an era of microservices and if you want to build one of that, ASP.NET is a great option. We will be using SQLite as our development database.
[02:30 - 02:58] It's very easy to install and it doesn't require to run any servers, which makes the development process very convenient. I know we can't use this as a production database, so we will be using Postgres as our production database. It is a very popular relational database service and most importantly, Heroku gives us an option to run Postgres database server for free , which means we can host our entire application on the internet without spending a single penny. How cool is that?
[02:59 - 03:10] And now since we are talking about deployment, let's see where we are hosting our project. Heroku provides some amazing deployment solutions and we will be deploying our application on Heroku.
[03:11 - 03:33] With our database deployed on Postgres, which again is provided by Heroku for free. I chose Heroku because it takes only few commands to take your application to the internet. It also gives us an option to create a free database. Now these are the main technologies. There is a lot more covered in this course. For that, let's move on with the course.