Implementing Generic Repository
In this lesson, we're going to implement generic repository to our application
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
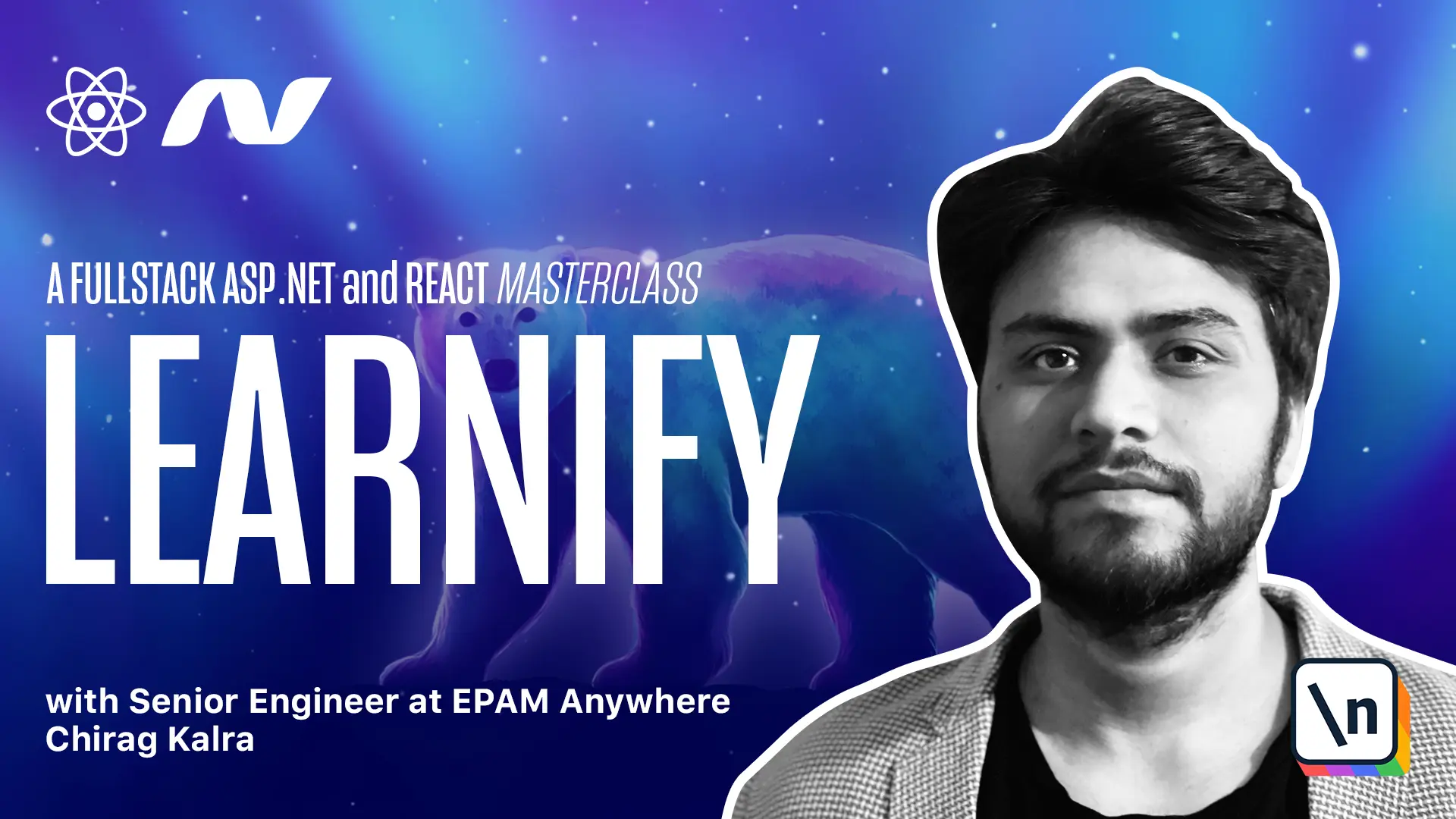
[00:00 - 01:34] We discussed how both of our repositories, courses and categories are doing the exact same job, to get list of data from our database and to get an individual entity from the database. It's not a problem when we have limited number of controllers, but when the project gets bigger, we write almost the same code all over again. If I open the interfaces, the only difference I see is the type. Here we are returning task iridonal list of category and here it is task iridonal list of course. And of course the type here is an integer and here is a good, but we can take care of it. What we can do is create a generic repository and what it will do is use a placeholder in place of type. So rather than using category, it will use a placeholder and when the code will compile, the generic placeholder will be replaced by the actual type. So it will be replaced by category here. And if you open the course repository, the type T will be automatically compiled to the course entity. We just have to specify the type T and everything else will be taken care of. So without wasting any further time, let's create our generically repository. I will create a new interface and we'll call it I generic repository. First of all, we will write our generic type T inside angle brackets here.
[01:35 - 01:46] T stands for type. You can call it anything really, but T for type sounds very straightforward. Now we just have to make two methods here. The first one returns iridonal list.
[01:47 - 03:36] So what we can do is write task of I read only list of type T, which will be replaced later on. And we can call it list all basin. And our second method will return the single entity and it will accept an id. So what we can do is write task of type T. We can call it get by id asin. And since our course repository and category repository have different types of id. So I can make it dynamic and id. Now we need to import task from system dot threading tasks. And I read only list from system dot collections dot generic. Now if you notice, we have just one repository, which is doing the same job as these two repositories. Let's do the implementation of this repository now. Inside the infrastructure project, we need to create a new class, which will be called generic repository . So I'll make a new class and we'll call it generic repository. This will derive from the i generic repository. So like i generic repository. And this will take dynamic type T and our generic type will be implemented in the generic repository as well. Let's import it using entity dot interfaces.
[03:37 - 04:04] Now we just have to implement this interface. So we'll go here and write implement interface. And the error is now gone. And if you remember, whenever we make a new repository and an interface, we have to register it to our starter class. So let's do that use command and P and click on startup.
[04:05 - 05:11] And we are here adding generic repository is a little different to register because we don't know the time at this time of registration. So what we will do is, anyway, we can write services dot add scoped and inside brackets, we need to write type of another bracket and i generic repository without T. And now it's the implementation, which is generic repository. So again, I will write type of another brackets, generic repository without T and just the angle brackets. And that's simply it. We have set up our generic interface. We have implemented it and we have registered it to our starter class. We just have to write these methods now. So let's do that in the next lecture.