The newline Guide to Modernizing an Enterprise React App
In this course, you'll learn all the pieces that go into modernizing an existing React application so it can take advantage of the latest framework features and run well for years to come.
- 5.0 / 5 (1 ratings)
- Published
- Updated
8 hrs 38 mins
46 Videos
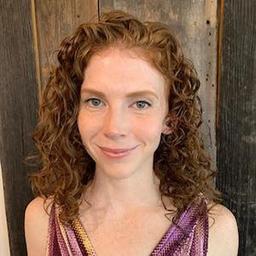
Paige Niedringhaus
Fullstack developer with a focus on frontend. In my day job as a staff software engineer for the IoT startup Blues Wireless, I use React and Hugo to build fun, beautiful, interactive applications.
In my spare time, I'm learning IoT programming with sensors, microcontrollers and Raspberry Pis.
01Remote
You can take the course from anywhere in the world, as long as you have a computer and an internet connection.
02Self-Paced
Learn at your own pace, whenever it's convenient for you. With no rigid schedule to worry about, you can take the course on your own terms.
03Community
Join a vibrant community of other students who are also learning with The newline Guide to Modernizing an Enterprise React App. Ask questions, get feedback and collaborate with others to take your skills to the next level.
04Structured
Learn in a cohesive fashion that's easy to follow. With a clear progression from basic principles to advanced techniques, you'll grow stronger and more skilled with each module.
How to modernize an existing React application according to today's standards
How React Hooks work compared to class components
How config tools Volta and Node engines simplify development
How to set up ESLint and Prettier to improve code quality
How to upgrade an existing React app and refactor the app as you go
How to write integration tests with React Testing Library to ensure app functionality
How end-to-end tests with Cypress can test mission critical user flows
How to incorporate a component library to speed up design and development time
In this course we'll dive into all the ways to modernize an existing React application - from tooling and linting, to testing and Hooks. React is a powerful, extremely popular JavaScript framework, but keeping applications up to date with all that it has to offer is tough - especially when dealing with the larger, enterprise-level business applications many developers are responsible for.
Why this course
The newline Guide to Modernizing an Enterprise React application will cover everything you need to know to confidently work on a React application of any size, in any state, and leave it better than when you found it.
While many React tutorials start with the latest version of React, or gloss over all the other things required to make sure apps vital to a company's success keep running, this course does not. In 54 lessons spanning 10 modules, it tackles head-on, the many things that must be considered when building and maintaining large, complex React apps.
Course modules
As you progress through this course, you'll gain a deeper understanding for how and why React Hooks came to be, the importance of various types of automated testing and project configuration, and how to leverage design systems to improve your application. You will gain a detailed understanding of how to modernize a React app in a real-world scenario, bringing it in line with today's development standards.
Part one: tooling and setup
The first part of this course will consist of getting familiar with our example application, and doing the behind-the-scenes work to set it up for success.
We'll learn about why React Hooks came about and how to use them, how to upgrade an outdated React app successfully, and how to use tools like Volta, ESLint and Prettier to improve the app's code and development experience.
- Module 0: Meet Hardware Handler - our outdated, but promising React application.
- Module 1: React Hooks - why they were created, the issues they solve and how the most common ones work.
- Module 2: Upgrading a React app - our app needs an upgrade to use Hooks, and we'll streamline development with Volta.
- Module 3: Project configuration - adding Prettier and ESLint to our app will dramatically improve code quality.
Part two: refactoring the code
With our app configured for success, we'll get down to the business of refactoring Hardware Handler.
I'll show you my strategy for converting class components to functional ones, and we'll go file-by-file keeping the app's functionality intact while upgrading it to use hooks - including custom hooks, and the Context API.
- Module 4: Classes to functions - we'll take every class component and turn it into a functional component.
- Module 5: Custom hooks - we'll identify where custom hooks can simplify our functional components further.
- Module 6: Context API - a few strategically placed React contexts will round out our code improvements.
Part three: testing
An updated application is only half the battle for enterprise-level apps; development teams must also be confident in their continued success as the apps expand in size and scope.
We'll add automated testing to our app using the widely accepted testing frameworks React Testing Library and Cypress.
- Module 7: Integration testing - the popular combination of Jest and React Testing Library will be used to unit test smaller pieces of our app.
- Module 8: End-to-end testing - the ease of writing e2es with Cypress Studio will blow you away.
Bonus: design systems
Finally, I've included a special bonus module about how to incorporate design systems into an already existing application, and switch out components in a systematic way. Design systems and component libraries are quickly gaining ground - especially in big orgs with lots of apps and development teams.
Being able to effectively use one is an important skill to learn.
- Module 9: Ant Design - we'll swap in the robust design system's components to handle our app's more complex interactions for us.
Bottom Line
In 10 modules, we'll go step-by-step taking an app from outdated in every way to up to today's high standards, giving you ample practice and hands-on examples along the way.
If you've been wanting to get more confident in your React skills and see what it takes to build enterprise apps in today's world, this is the course for you.
Our students work at
Sample Course Lessons
Course Syllabus and Content
Introduction
2 Lessons 37 Minutes
- Sneak Peek00:21:20
- Sneak Peek00:15:57
React Hooks
8 Lessons 39 Minutes
- Sneak Peek00:01:16
- Sneak Peek00:10:37
- Sneak Peek
- Sneak Peek
- Sneak Peek00:10:47
- Sneak Peek00:13:31
- Sneak Peek
- Sneak Peek00:03:31
Upgrade the React App
4 Lessons 11 Minutes
- Sneak Peek00:00:51
- Sneak Peek
- Sneak Peek00:08:03
- Sneak Peek00:02:29
Configure Prettier and ESLint
4 Lessons 34 Minutes
- Sneak Peek00:01:25
- Sneak Peek
- Sneak Peek00:29:48
- Sneak Peek00:02:47
Refactor React Classes to React Hooks
7 Lessons1 Hours 16 Minutes
- Sneak Peek00:01:05
- Sneak Peek00:05:52
- Sneak Peek00:13:45
- Sneak Peek00:16:06
- Sneak Peek00:18:31
- Sneak Peek00:20:17
- Sneak Peek00:01:16
Create Custom Hooks for the App
5 Lessons 48 Minutes
- Sneak Peek00:00:55
- Sneak Peek00:12:49
- Sneak Peek00:11:41
- Sneak Peek00:20:55
- Sneak Peek00:02:18
Incorporate the Context API
4 Lessons 35 Minutes
- Sneak Peek00:00:57
- Sneak Peek00:18:23
- Sneak Peek00:14:15
- Sneak Peek00:02:07
Integration Testing with Jest and React Testing Library
7 Lessons1 Hours 47 Minutes
- Sneak Peek00:02:16
- Sneak Peek00:07:46
- Sneak Peek00:18:53
- Sneak Peek00:52:34
- Sneak Peek
- Sneak Peek00:23:05
- Sneak Peek00:02:56
End-to-End Testing with Cypress
6 Lessons 47 Minutes
- Sneak Peek00:01:36
- Sneak Peek
- Sneak Peek00:13:31
- Sneak Peek00:28:45
- Sneak Peek
- Sneak Peek00:04:03
Bonus Module: Add a Design System Library
6 Lessons1 Hours 16 Minutes
- Sneak Peek00:01:44
- Sneak Peek00:06:03
- Sneak Peek00:14:44
- Sneak Peek00:22:19
- Sneak Peek00:29:10
- Sneak Peek00:02:52
Summing It All Up
1 Lesson 2 Minutes
- Sneak Peek00:02:20
Subscribe for a Free Lesson
By subscribing to the newline newsletter, you will also receive weekly, hands-on tutorials and updates on upcoming courses in your inbox.
What Students are Saying
Meet the Course Instructor
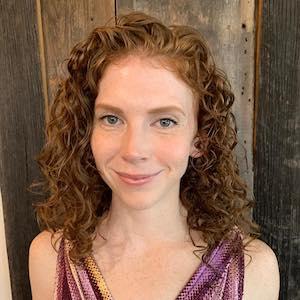
Purchase the course today
newline Pro Subscription
$18/MO
Get unlimited access to the course, plus 60+ newline books, guides and courses. Learn More
Billed annually or $40/mo billed monthly. Free to cancel anytime.
- Discord Community Access
- Full Transcripts
- Money Back Guarantee
- Lifetime Access
Plus:
- Unlimited access to 60+ newline Books, Guides and Courses
- Interactive, Live Project Demos for Every newline Book, Guide and Course
- Complete Project Source Code for Every newline Book, Guide and Course
- Best Value 🏆
Frequently Asked Questions
Who is this course for?
Intermediate to advanced developers already comfortable with React and somewhat familiar with the React framework but not necessarily well-versed with the newest syntax like React Hooks. Also, for anyone who wants to learn the kinds of things that go into large, long-lived enterprise-sized React apps: the tooling and configuration, testing, design systems and strategies for updating existing apps to keep them fresh and relevant
I already know how to use Hooks, will I still get something out of this course?
You sure will. This course goes way beyond React Hooks because the React apps supporting big companies encompass way more than that. We cover integration and end-to-end testing, project tooling and configuration like Volta, Prettier and ESLint, design system library integration and more. You may know React, but there's a lot more to it than just Hooks
What if I need help?
You can ask us questions anytime through the community Discord channel #modernizing-an-enterprise-react-app or by sending us a message.