Creating React Libraries from Scratch
In this course we'll cover what it takes to maintain, write, and publish a React library from scratch.
- 4.5 / 5 (4 ratings)
- Published
- Updated
1 hr 6 mins
19 Videos
01Remote
You can take the course from anywhere in the world, as long as you have a computer and an internet connection.
02Self-Paced
Learn at your own pace, whenever it's convenient for you. With no rigid schedule to worry about, you can take the course on your own terms.
03Community
Join a vibrant community of other students who are also learning with Creating React Libraries from Scratch. Ask questions, get feedback and collaborate with others to take your skills to the next level.
04Structured
Learn in a cohesive fashion that's easy to follow. With a clear progression from basic principles to advanced techniques, you'll grow stronger and more skilled with each module.
Creating a React library from
yarn init
to deployed on npmThe different types of JavaScript dependency types and versioning
Setting up documentation for contributers and consumers
Keeping up with code quality while multiple people work on a project
Different JavaScript module systems
In this course, we'll cover what it takes to write, maintain, and publish a React library from scratch. In the 2020 State of JS survey, an annual survey conducted of the frontend JavaScript community, React came in as the most used framework for frontend development. Because React is so popular, building libraries in it can open you up to a wide range of users, contributors, and possibilities!
Why this course
Building a library is so much more than just the code. We need to know the ins and outs of versioning, deploying, building, documenting, dependency management, module types, tooling, and more. This is a daunting task for just one tutorial to cover. Creating React Libraries from Scratch will teach you everything you need to know to succeed in creating a library.
Don't worry if you're not a React wizard. This course is designed for beginner/intermediate developers who have never deployed a library before and have minimal React experience. However, you should be familiar with JavaScript.
Course Topics
Throughout Creating React Libraries from Scratch we'll develop a React Hook called Scroller that will provide a utility for scrolling around web pages—taking advantage of window.scrollTo. We'll start in module 1 with an empty directory; adding documentation files, installing dependencies, and setting up GitHub. Right away, you'll have a project that could be deployable as a fully functional library! In module 2 we'll implement scroller while taking advantage of Storybook to visually test our code. By module 3 Scroller will be deployed to npm in multiple JavaScript module formats. Finally, in module 4, we'll look at different tools and techniques in software development to maintain a library. This includes linting, unit tests, TypeScript, and Lint-Staged! By the end of this course, you'll be able to run npm install scroller, and take advantage of React code you wrote in future projects.
Creating React Libraries from Scratch will dive into:
- initializing a new npm package
- maintaining code quality
- publishing to npm
- keeping contributors on track
- exposing modules that work in NodeJS and the Web
- unit-testing
- Storybook for demoing components
- exposing typing using TypeScript
- and more!
Our students work at
Course Syllabus and Content
Initialize
4 Lessons 18 Minutes
- Sneak Peek00:05:25
- Sneak Peek00:04:36
- Sneak Peek00:03:07
- Sneak Peek00:05:11
Implement
3 Lessons 7 Minutes
- Sneak Peek00:01:07
- Sneak Peek00:02:52
- Sneak Peek00:03:56
Deploy
4 Lessons 15 Minutes
- Sneak Peek00:02:18
- Sneak Peek00:04:54
- Sneak Peek00:02:54
- Sneak Peek00:05:34
Maintain
5 Lessons 19 Minutes
- Sneak Peek00:00:43
- Sneak Peek00:07:01
- Sneak Peek00:04:33
- Sneak Peek00:04:33
- Sneak Peek00:02:23
Summary
1 Lesson 34 Seconds
- Sneak Peek00:00:34
Subscribe for a Free Lesson
By subscribing to the newline newsletter, you will also receive weekly, hands-on tutorials and updates on upcoming courses in your inbox.
What Students are Saying
Meet the Course Instructor
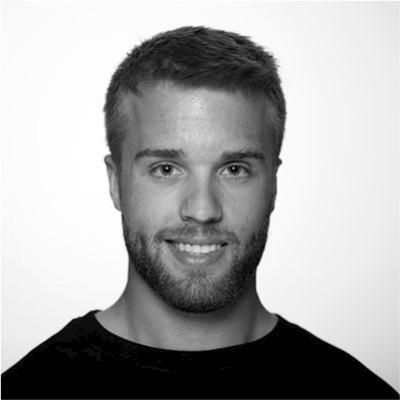
Purchase the course today
newline Pro Subscription
$18/MO
Get unlimited access to the course, plus 60+ newline books, guides and courses. Learn More
Billed annually or $40/mo billed monthly. Free to cancel anytime.
- Discord Community Access
- Full Transcripts
- Money Back Guarantee
- Lifetime Access
Plus:
- Unlimited access to 60+ newline Books, Guides and Courses
- Interactive, Live Project Demos for Every newline Book, Guide and Course
- Complete Project Source Code for Every newline Book, Guide and Course
- Best Value 🏆
Frequently Asked Questions
Who is this course for?
The entry-level or intermediate developer with some knowledge of React or Web Dev, who has never published an npm package.
What if I need help?
You can ask us questions anytime through the community Discord channel or by sending us a message.