Using React Higher Order Components to Frame a Canvas Editor
Making graphic list items clickable; and the editor(detail) route. In the process, we'll learn how to use React's Higher Order Components with Reagent.
This lesson preview is part of the Tinycanva: Clojure for React Developers course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Tinycanva: Clojure for React Developers, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
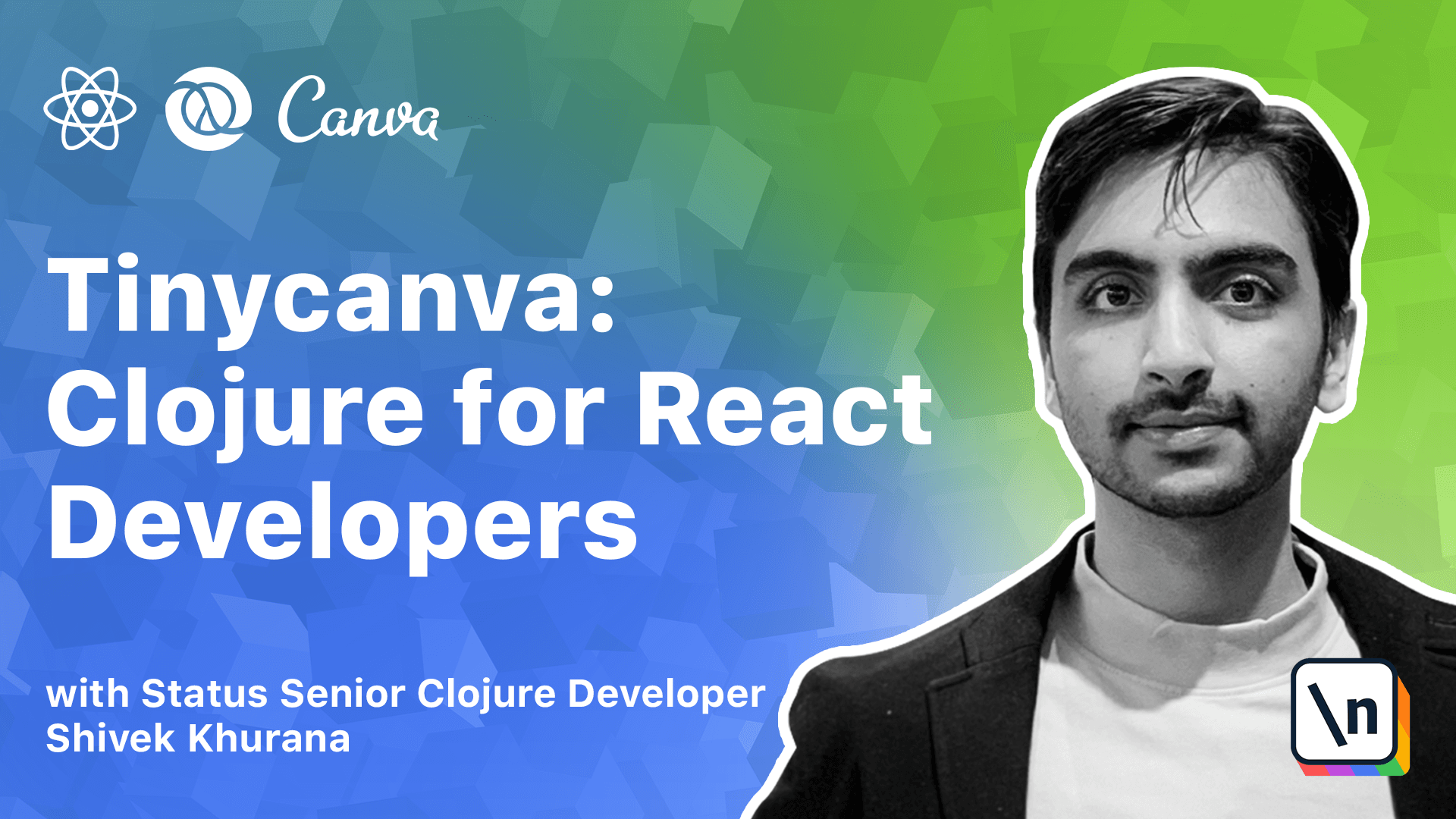
[00:00 - 00:10] With the graphics list in place, we can now build the details page for each graphic. The details page will include an editor component that will let us draw the actual graphic.
[00:11 - 00:23] In this chapter we will focus on the routing aspect and not concern ourselves with the actual editor. React Router provides various mechanisms to parse route params which is graphic ID in our case.
[00:24 - 00:37] There is a higher order component based approach and we can use the vid router at your C and also take a hooks based approach using use params hook. We are going to use vid router at your C in our app.
[00:38 - 00:47] You can use hooks too but reagent support for hooks is limited as of this writing. The vid router at your C expects a React component as an argument.
[00:48 - 00:57] One tiny issue with React components is that ratams don't work with React. The ratams work fine as children of React components.
[00:58 - 01:09] The solution is to create a transparent trapper to the desired reagent component and parse the props in manually. Then reactify this wrapper component so ratams can continue to work.
[01:10 - 01:18] Let's understand this with an example. We define the counter atom and the component x page that depends on this counter atom.
[01:19 - 01:31] Now if we reactify this component and render it at a route, the counter will stop working. To bypass this we can create an x page container which just renders the x page component with the props.
[01:32 - 01:44] Now if we reactify x page container, the counter atom will continue to work in x page. The details for a graphic page will be available at graphics/graphic_id route.
[01:45 - 01:59] As per our app structure, the namespace responsible for this route is app.pages .graphics.detail. We will also need an additional switch component in app.pages.graphics since we need to compose routes as follows.
[02:00 - 02:10] Let's update our app.pages.graphics namespace to accommodate this switch. We extracted the existing page component to list component, replaced page with the switch.
[02:11 - 02:27] When the route path is graphics, the list component will be rendered and when the route path is graphics/graphics_id, the graphics detail container will be reactified and rendered. It will also receive route properties, courtesy of a router/huc.
[02:28 - 02:39] Graphic detail container internally renders the detail page which we haven't created yet. The exact drop prevents the list component from being re-rendered on graphics/g raphic_id.
[02:40 - 02:47] This is how React Router composes. The graphic card component should redirect to graphics/graphic_id.
[02:48 - 02:54] We can achieve this by using React Router's link component. Let's update our card and wrap its content in a link.
[02:55 - 03:00] The id pass to the graphic is a keyword. The name function converts a keyword into string.
[03:01 - 03:15] If the id of graphic was string -mxfh42, then the id argument will be keyword m xfh42. And the 2 prop will be graphics/hfh42.
[03:16 - 03:32] If the name function was not used, the 2 prop would have been the string -golin -mxfh42 which might have caused issues with parsing. We can now populate the app.pages.graphics.detail and realize our wireframe.
[03:33 - 03:39] We require the packages and namespaces we need for this component. Let's create the page next.
[03:40 - 03:46] The props will be a map with keys, location, match and history. A param's map will be present in the match object.
[03:47 - 04:00] The key values of param's map will be a chase object of parse throughout parameters. Since we are on the graphics/graphic id route, the parsed param's chase object will contain graphics id.
[04:01 - 04:17] Go.object/get value by keys is a gcp function and is equivalent to get in for js objects. If you have a nested js object like a, b, c, the get value by keys object a, b will return c.
[04:18 - 04:25] When we have the graphic id, we subscribe to app.db to get the graphic with that id. We will write this subscription zone.
[04:26 - 04:38] When the graphic is available, id route is parsed and the subscription is realized, we can render the actual component. The nav component is not global navigation, but local navigation as shown in the wireframe.
[04:39 - 04:46] We also render the string version of graphic object so we can see things in action. Graphic by id subscription.
[04:47 - 04:53] The subscription is similar to deleting graphic id. It accepts a graphic id and returns a value for that id.
[04:54 - 05:04] This navigation bar has two purposes. To link back to the home page and to show the name of the graphic, we can use blueprint snap bar component again to set this up.
[05:05 - 05:16] Since the snap is only used by graphics/detail page, we can place it inside app .pages.graphics.detail. This nav is similar to the global nav we defined earlier.
[05:17 - 05:26] We haven't used the graphic id, but we will need it to update the name in later sections. The nav bar is blue this time, but feel free to choose your own colors.
[05:27 - 05:41] With everything in place, you should be redirected to the details page when you click on a card and back home when you click on the home icon. In this chapter, we used the width router HUC to capture route perhams and created the details page.
[05:42 - 05:45] We also updated the graphics card to redirect to the detail.