What is Syntaxlessness? How to Write More Expressive Code
C-style languages invisibly impose a syntax, whereas Lisps do not. This makes Lisps more expressive and easier to grasp.
This lesson preview is part of the Tinycanva: Clojure for React Developers course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Tinycanva: Clojure for React Developers, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
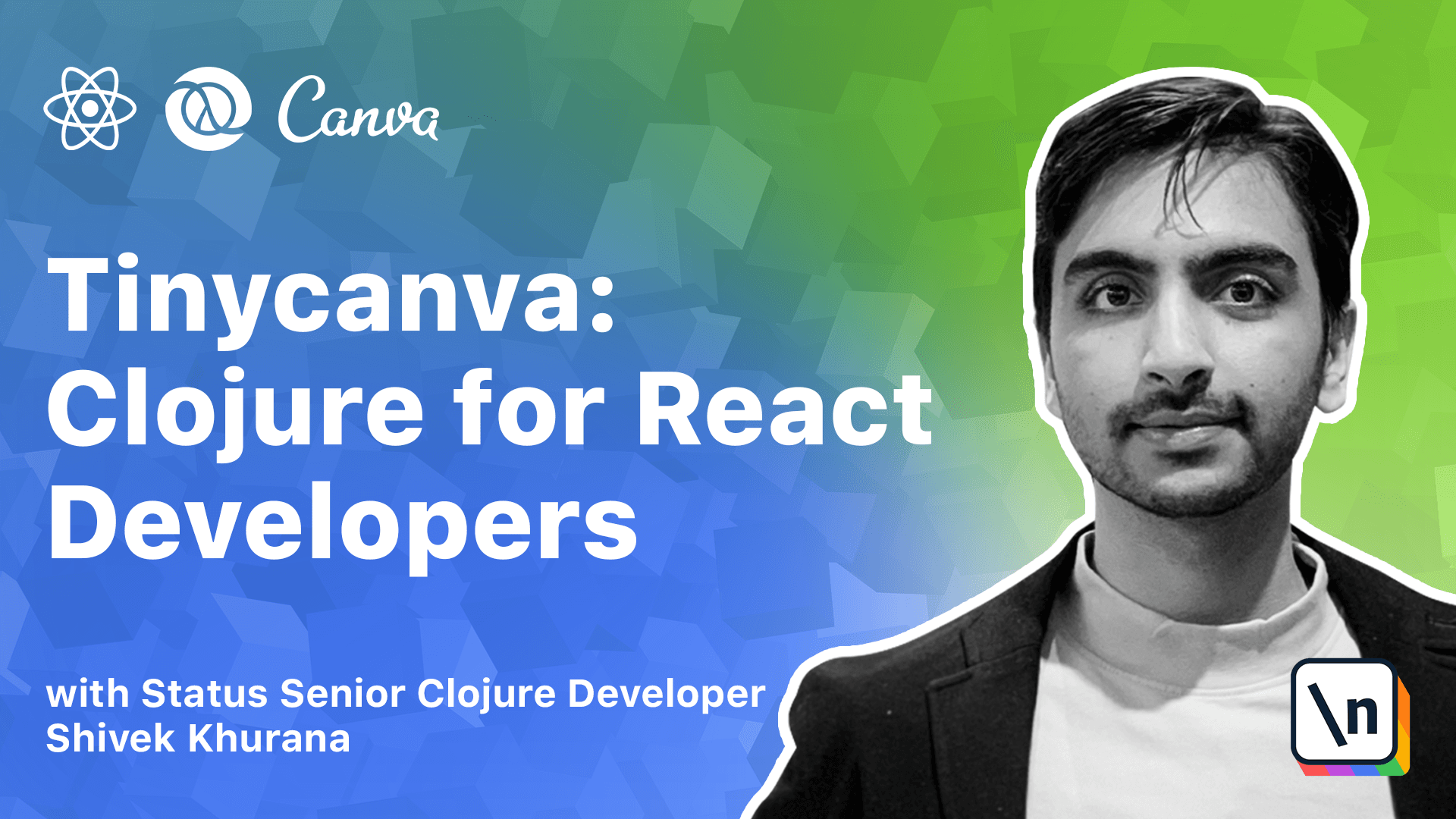
[00:00 - 00:07] In the last chapter we studied about closure syntax. In this chapter, I will try to convince you that closure does not have a syntax .
[00:08 - 00:18] I know it sounds a little odd, but please bear with me for a few minutes and things will make sense. Consider this simple JavaScript if condition.
[00:19 - 00:30] The keywords if and else and the curly brackets have to be in specific positions for this code to execute properly. This constitutes the syntax of the language.
[00:31 - 00:40] The same condition enclosure can be written as follows. On the surface the two might look similar, but there are many underlying differences.
[00:41 - 00:57] In JavaScript, the positioning of the keywords if and else and the curly brackets are a part of the syntax. Other operations like defining a function, defining a tri-catch block, defining a variable will have a difference in tax in JavaScript.
[00:58 - 01:16] But in closure, if is just a function call and so is every other operation you can perform. The only rule that you need to keep in mind is that a list starts with parentheses and the first element of the list is an operation and all other elements are the arguments to that operation.
[01:17 - 01:26] In that sense closure is way more expressive than a C family language. We can rewrite the if conditional in closure as follows.
[01:27 - 01:36] Notice how we moved the function if inside the print function. Now you might be wondering that you can do the same thing in JavaScript using the ternary operator.
[01:37 - 01:49] That's a great observation, but we'd like to point out that the ternary operator is an additional piece of syntax. With closure it was just a function call, but ternary operator is an escape hook.
[01:50 - 02:00] It is not as extensible as composing functions would be. If JavaScript was as expressive as closure then something like this would have been possible, but it is not.
[02:01 - 02:17] The keywords if and else only work in certain positions, you can't compose them freely. Closure functions can be nested at multiple levels and as long as your code is a valid list, there is no other syntactical rule that you need to follow.
[02:18 - 02:19] hence, syntaxlessness.