Module 7 Summary
This lesson is a summary of the work done in Module 7.0.
This lesson preview is part of the TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL with a single-time purchase.
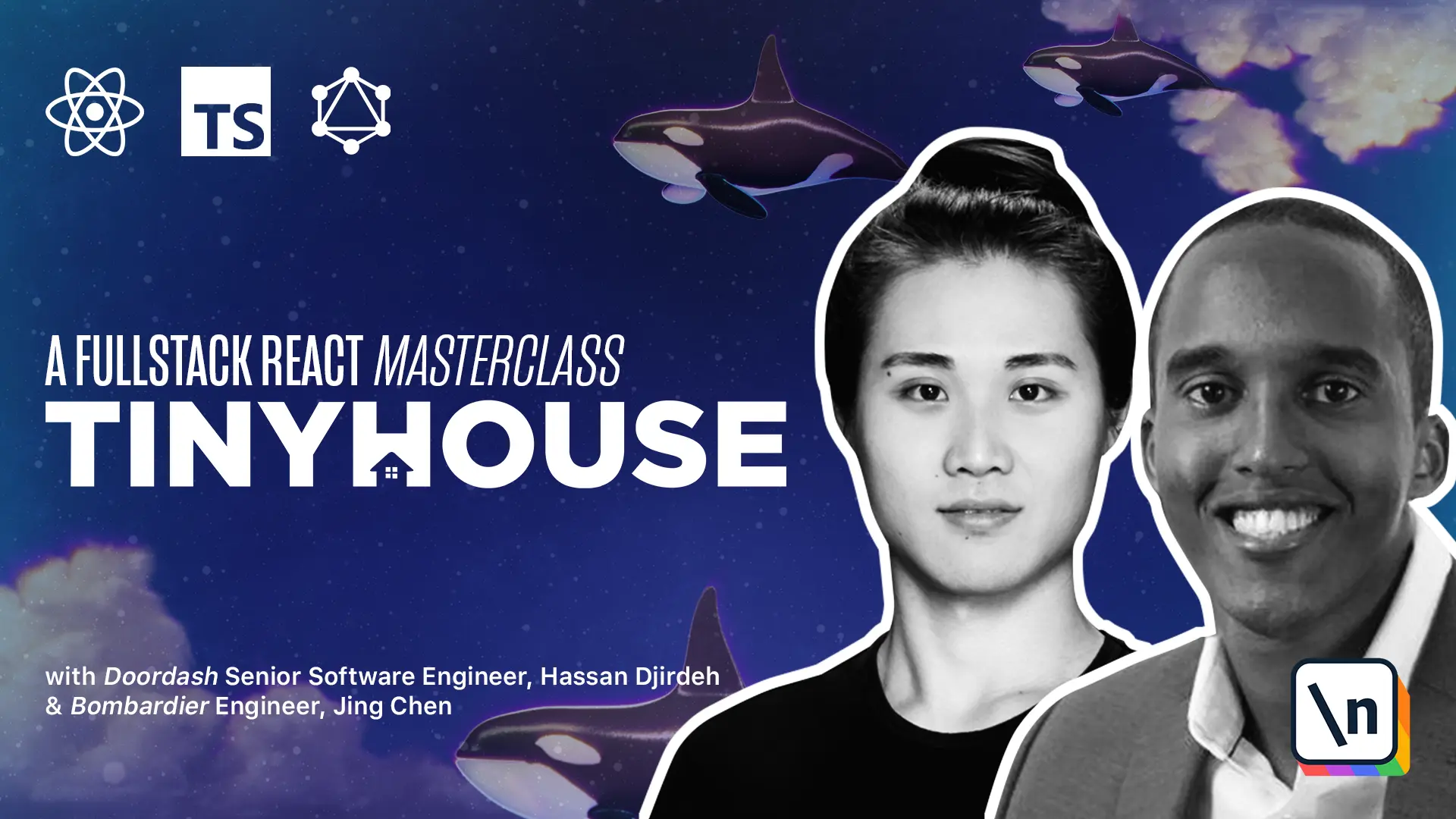
[00:00 - 00:13] In this module, we've introduced a few different hooks to allow us to manipulate and handle information in our listings component. By the end of the module, we created and used our own custom hooks.
[00:14 - 00:27] They use query hook and they use mutation hook. The use query hook is responsible in consolidating the information and having a GraphQL query be run the moment the component mounts.
[00:28 - 00:44] It returns a series of information, such as the data that is to be returned from the query, as well as the loading and error status of the query request. It also returns a refetch property, which allows components to refetch a query whenever a component may want to.
[00:45 - 01:09] To keep track of the state of information within our query, we used React's use reducer hook. Initially, we used the use state hook, which allows us to add React State to functional components. We moved to another hook that does the same, use reducer, to get a clearer separation between updates to the state object and actions needed to update the state object.
[01:10 - 01:29] The use effect hook is used to invoke the query request the moment the component mounts. To be able to extract the function needed to make the request outside of the use effect hook, we used the callback hook to memoize the fetch function to never run until the query parameter is changed.
[01:30 - 01:46] Query is unlikely to ever change, since it's to be a constant value declared outside of the components. But in this case, this satisfies the use effect requirements to ensure that values are never referenced as the stale value beforehand.
[01:47 - 02:05] To better handle errors from our GraphQL request, we check for two error scenarios. One where the actual request completely fails, that is to say has an error status code, and the other is where the request is made, but our GraphQL API returns errors within the errors field of the request.
[02:06 - 02:27] The use mutation hook is similar to the use query hook except for where a use effect isn't being used to make the request when the component first mounts. The listings component uses the use query and use mutation hooks at the top of the component, and it de-structs the values that are needed from each of the hooks.
[02:28 - 02:49] Each of the values here are being used to determine what is to be displayed in the UI of the listings components. Though we're able to proceed with what we've done to build a larger application , there are some limitations to just making simple fetch commands to retrieve data from a GraphQL API.
[02:50 - 03:13] For example, assume we had numerous components, and when a component like listings is first rendered, we make a GraphQL query. When we navigate elsewhere and remount this component again, it'll be nice to have the data we've already queried cached in our UI, instead of having to make a repeat query to get the same data that we may already have.
[03:14 - 03:30] Our use query hook aims to make a query the moment a component mounts, but what if we wanted to make a query by invoking an action only, like clicking a button , entering a form, etc. Should we use the use mutation hook instead for this?
[03:31 - 03:47] What if we wanted some more complicated functionality with how we handle our GraphQL requests? An example of this could be, what if we were able to refetch another query based on a fact that a certain query or mutation has been made?
[03:48 - 04:05] Assume that when we delete a listing, let's just say we wanted to re-query a user query that provides information in the same page. We could try and tailor what we've done here to make this happen, but this is when things start to get a lot more complicated.
[04:06 - 04:24] This is where the Apollo client, or in our instance, the React Apollo library fits in. From the Apollo GraphQL documentation, Apollo client is a complete state management library for JavaScript apps.
[04:25 - 04:39] It allows us to fetch data and structure our code in a predictable, declarative way that's consistent with modern React practices. Some of the features that it has is declarative data fetching.
[04:40 - 04:53] Excellent developer experience is designed for modern React by allowing us to use hooks. It's incrementally adoptable, it's universally compatible, and it's community driven.
[04:54 - 05:04] In the next module, we're going to install the React Apollo library, and we're going to use the hooks given to us from React Apollo in our listings components .