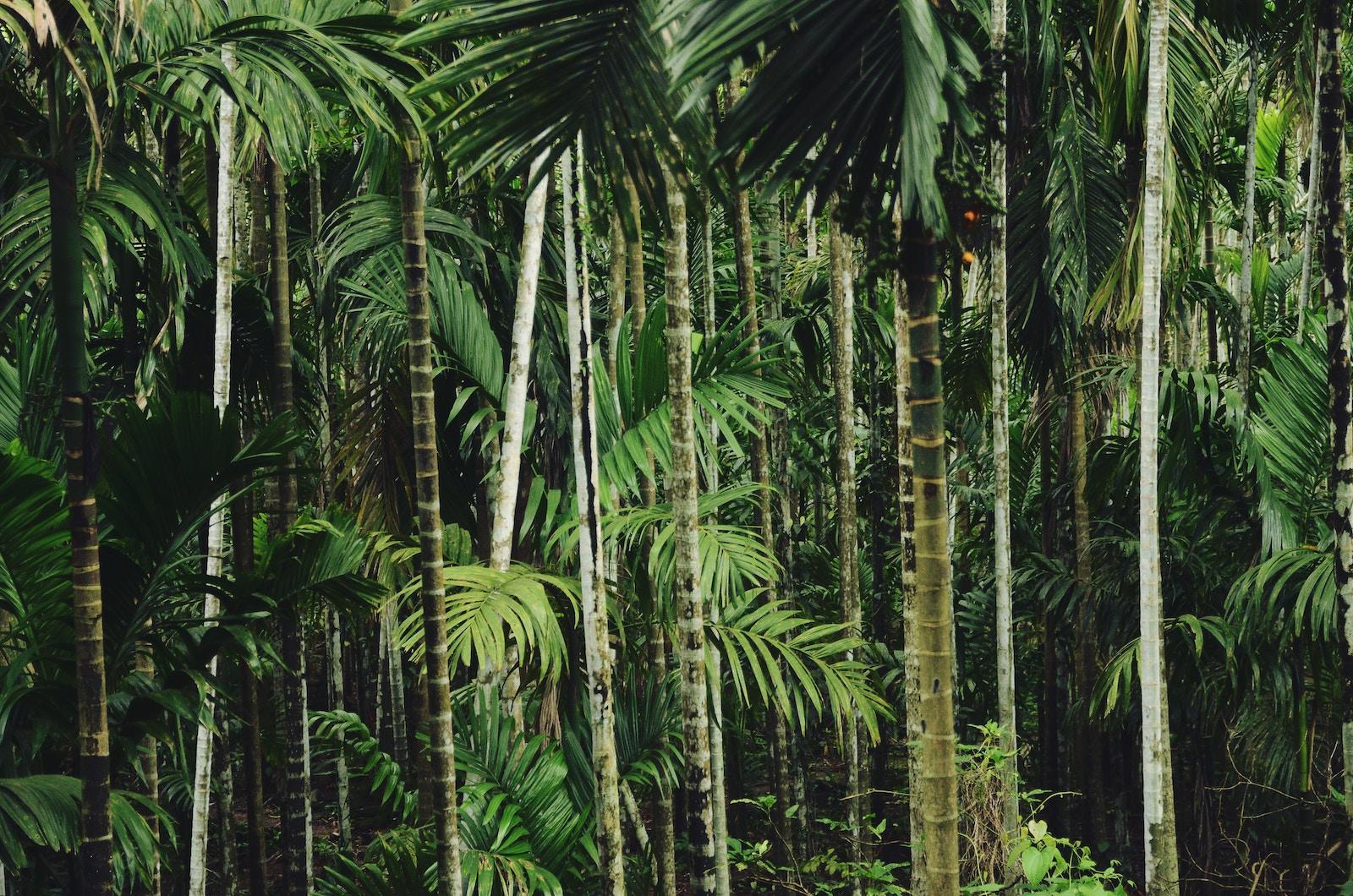
30 Days of React Native
First component
This post is part of the series 30 Days of React Native.
In this series, we're starting from the very basics and walk through everything you need to know to get started with React Native. If you've ever wanted to learn React Native, this is the place to start!
First component
Now that we'll all set up, let’s dive into how components work in React Native.
If you’re already familiar with React, then you already know how to write in React Native. This is because they both share a core concept - using components to construct interfaces.
If you’ve never used React for the web before, there’s no need to worry! Let’s dive into how components work in React Native.
Component structure
When we create a new application with Expo, a single component is created by default in App.js
.
Let’s step through each part of the file. All our imports are declared at the very beginning:
import React from "react";
import { StyleSheet, Text, View } from "react-native";
Just like writing components in React for a web page, we need to import the react
library in order to be able to create our components. In the second line however, a number of core APIs from React Native are also imported:
StyleSheet
: API used to set styles outside of the component functionText
: Used to display text (analogous to the element in HTML)View
: Used to define layouts and draw boxes with borders (analogous to the element in HTML)
On the web, core elements such as div
and span
never need to be imported and are supported by every browser automatically. In React Native however, every component must be imported.
Every core React Native API will be explored in more detail later in the series.
There are two ways to define a component using React Native (and React):
- A function component that uses a JavaScript function
- A class component that uses an ES6 class
Underneath our imports we can see our component defined as a function:
export default function App() {
return (
<View style={styles.container}>
<Text>Open up App.js to start working on your app!</Text>
</View>
);
}
The function returns what we want to render within that part of our application. In this example, we have a single View component that wraps a Text component that displays a simple message.
This component can also be written using a class instead:
export default class App extends React.Component {
render() {
return (
<View style={styles.container}>
<Text>Open up App.js to start working on your app!</Text>
</View>
);
}
}
Defining a component using a class requires extending React.Component
. Using extends
allows us to declare a class as a subclass of another class. In this example, App
is a subclass of React.Component
.
React Native reads both of these components in the exact same way. However, using class components make it possible to define state or use lifecycle methods.
Hooks, a newer React feature, also makes it possible to use state and lifecycle operations within function components
The last piece of our component is our style definitions:
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center"
}
});
The StyleSheet API is used here to define a container
style that sets background color and a few flexbox properties. Using flex: 1
makes the View
expand to fill the entire screen. The combination of alignItems: center
and justifyContent: center
ensure the text component is aligned in the vertical and horizontal center.
Ready to learn more about styling in React Native? We’ll be exploring this in more detail in tomorrow’s article!
The entire source code for this tutorial series can be found in the GitHub repo, which includes all the styles and code samples.
If at any point you feel stuck, have further questions, feel free to reach out to us by:
- Creating an issue at the Github repo.
- Tweeting at us at @fullstackio.
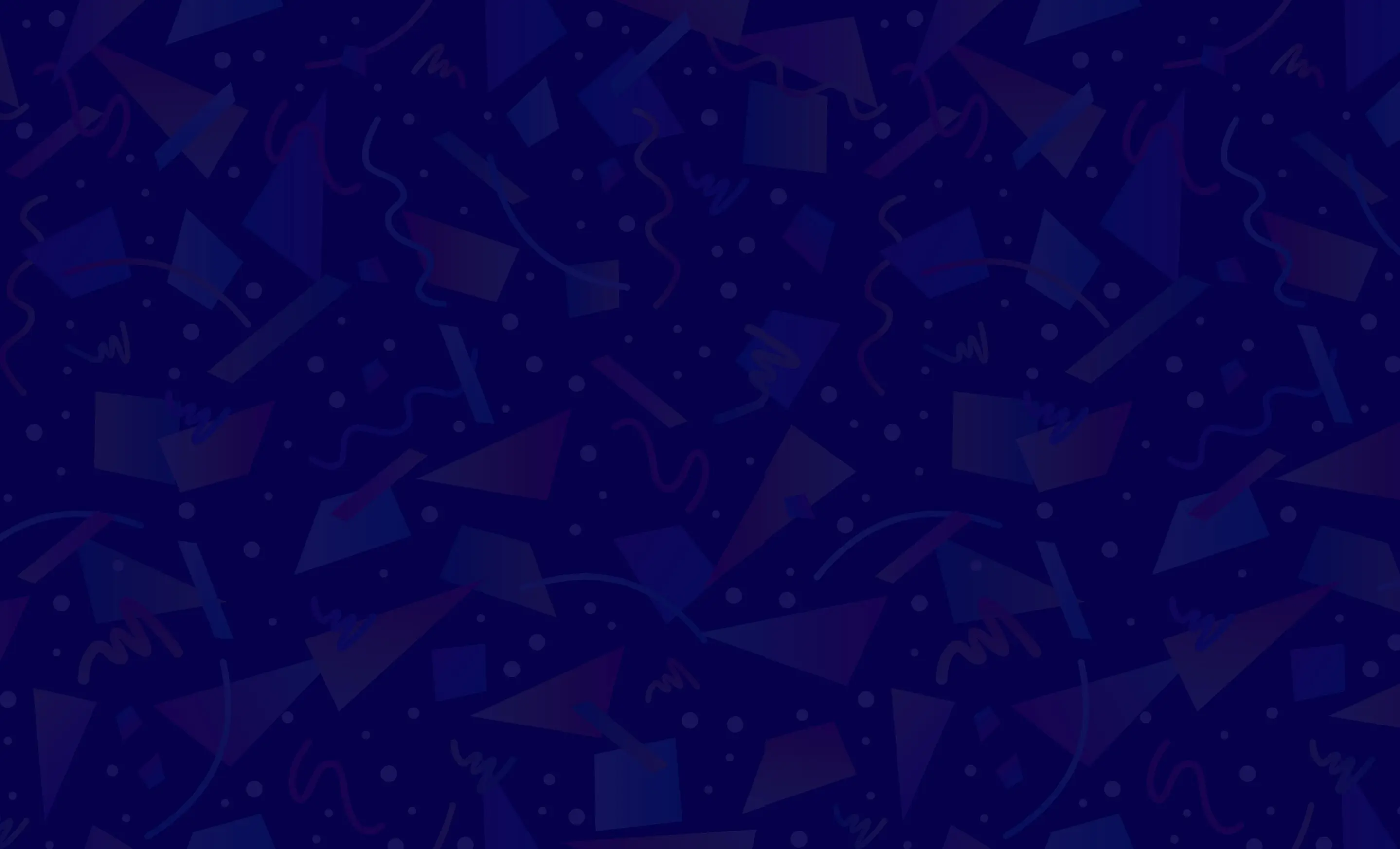
Get started now
Join us on our 30-day journey in React Native. Join thousands of other professional React Native developers and learn one of the most powerful mobile application development frameworks available today.