How to Build a Split Pane Layout in React
The layout challenge that is as old as the web itself is putting two items next to each other. In this lesson, you will learn how to build the Split primitive to solve this problem.
This lesson preview is part of the Composing Layouts in React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Composing Layouts in React, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
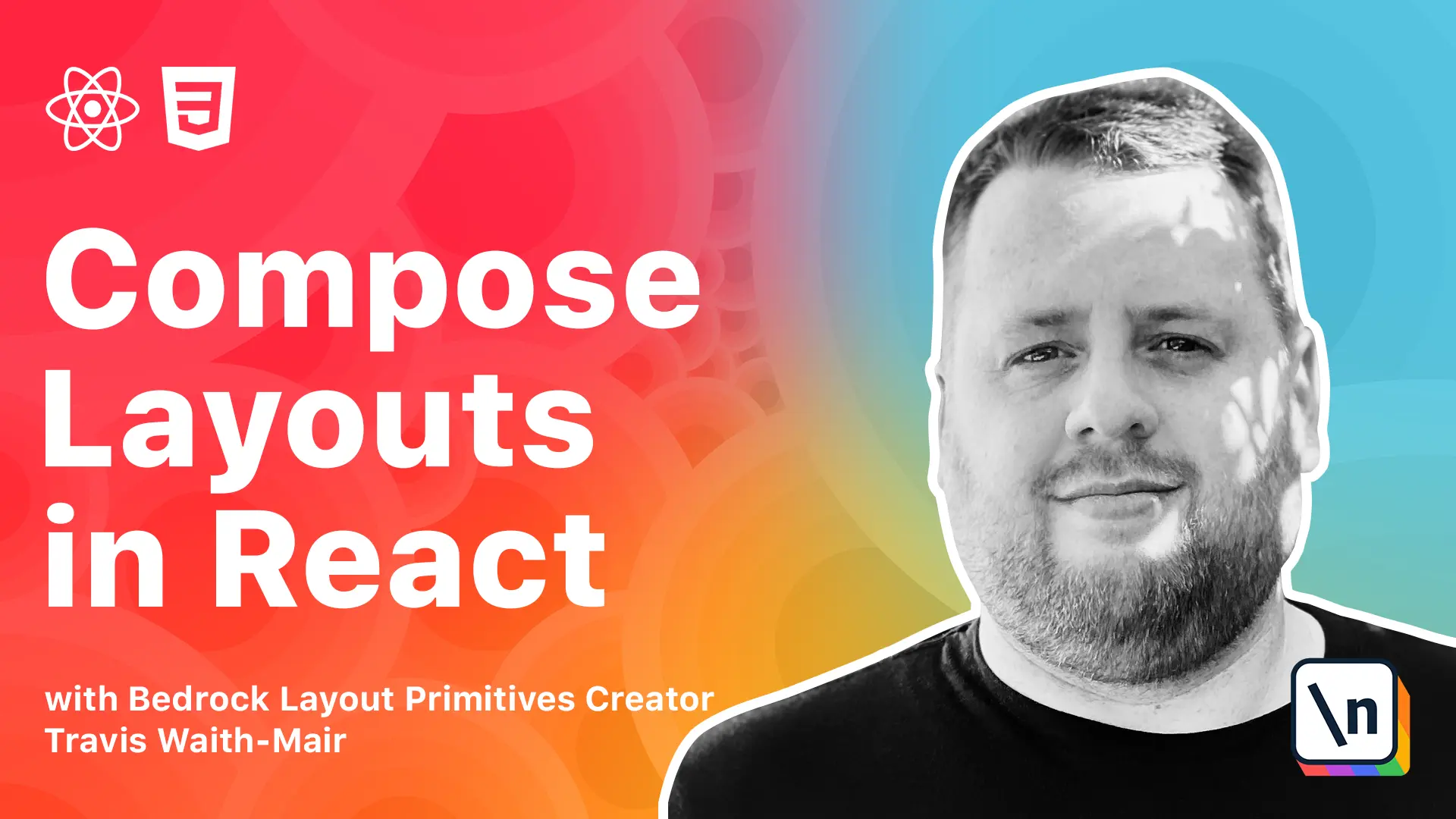
[00:00 - 00:09] Hey everybody, Travis here with another lesson in composing layouts and React. In this lesson we will learn about the split.
[00:10 - 00:20] For the longest time floats were the go-to tool for putting two things next to each other. Unfortunately since this was not what floats were designed for, this created as many problems as it solved.
[00:21 - 00:30] Luckily modern CSS makes it so much easier to solve. In this lesson we are going to build this widget.
[00:31 - 00:37] It's the form sidebar. Now similar to the stack primitive, we want a gutter in between the two parts.
[00:38 - 00:48] Unlike the stack component we need to be able to split the two children into fractional parts. In this case a one third by two third split.
[00:49 - 01:03] Okay just like in the last lesson, you can follow along by clicking on the starter project in the link below under the solution heading. But yeah let's start with some basic markup.
[01:04 - 01:18] So we always want to import React from React. And in this example we are using a form component.
[01:19 - 01:31] Don't worry about this form component, we will go more into that in the next lesson. But let's import that in.
[01:32 - 01:52] Form. And through the power of copy and paste, let's go ahead and bring that in.
[01:53 - 02:24] Oops, let's not do this. Let's freeze this.
[02:25 - 02:59] Okay let's try that again. Okay yeah we wait just like last time.
[03:00 - 03:14] We are going to start with a starter here, you can call it split.jsx. But if you don't want to you can just change that and just make sure you just change it on line eight here on the index.
[03:15 - 03:31] And just like everything we're going to take advantage of the copy and paste functionality just to get things started. Now once again we need import react from React.
[03:32 - 03:53] We also are going to import a component from this form.js file over here. The only reason I have this is just so we don't focus on the form part.
[03:54 - 04:03] We're really just worrying about splitting this section and this section from each other. We'll go more into this form component in the next lesson.
[04:04 - 04:13] But for now just know that it's wrapped in a div and that there's a bunch of input groups inside of it. And that's all you need to worry about for now.
[04:14 - 04:29] So we're going to import that form and let's refresh the page. We have an error.
[04:30 - 04:48] Oh yeah and it also helps to acknowledge that the form is a default not named in my import so we all make mistakes here. So there we go.
[04:49 - 04:58] This is how it looks personal information. The information you will provide will be displayed publicly and then our form.
[04:59 - 05:24] Now knowing that the H2 and the span will both need to be a sibling of the form component. I just kind of had it preemptively wrapped them in a div and then the outer div is what we're going to use to eventually control that split.
[05:25 - 05:47] Now let's go ahead and start building our split primitive. So once again we bring in styled and with a little hump the magic of copying paste let's go ahead and bring this in.
[05:48 - 06:00] Now the other thing we're going to bring in is that spacing map from the split lesson. I've kind of had to put that in a separate file just to make things easier and keep things clean.
[06:01 - 06:20] So let's go ahead and bring that in. Let's get this is a name to export this time so let's go ahead and do that.
[06:21 - 06:29] And there we go. This you've already seen so I'm not going to go into it.
[06:30 - 06:45] This is exactly what we did with the stack primitive and same thing with display grid. But this time we are doing something a little differently.
[06:46 - 06:56] We are actually defining some columns here. Now the grid template columns property allows you to define how many contracts we have and how wide they should be.
[06:57 - 07:06] We define the width of each contract using any valence CSS unit. It could be anything like 30 pixels, 50 percent and two rams.
[07:07 - 07:27] And the number of columns are defined by how many we put in here with a space in between them. So let's say we wanted to have three columns of 30 pixels, 50 percent and two r ams.
[07:28 - 07:43] Well then we would do 30 pixels, 50 percent and two rams. And this would create three column tracks of exactly those sizes.
[07:44 - 08:01] So you'll notice what I had in here was one FR and one FR. FR is a special unit only available to the grid if you're using display.grid.
[08:02 - 08:17] Display grid is a unique size unit that is sometimes called the fraction. And it basically says give me one fraction of the remaining space available.
[08:18 - 08:29] So in this case we're saying hey this first column I wanted to have one fraction and this column to have another fraction. And because they're the same amount it's basically going to split the columns.
[08:30 - 08:38] It's going to take that remaining space and divide it among the two columns. Which turns out to be a 50/50 split.
[08:39 - 08:48] So we can see that here. We wrap everything in a split instead of the div that we had.
[08:49 - 09:07] And already we see that this personal information section is now on to the left and our form is on the right. Now it is important to understand that this is an FR unit is not the same thing as a percentage.
[09:08 - 09:36] However when we use percentage if we were to say like 50% 50% we are saying give me 50% of the size of the parent. But that doesn't take into account that we have a gap property on here of one RAM by default.
[09:37 - 10:01] So now we made this column 50% of the parent and this 50% of the parent and we 're flowing over by one RAM because we didn't take into account this one gap. That's where the FR unit really shines is it knows how many how much space is available after all is said and done.
[10:02 - 10:27] And you won't get these overlaps like we had with the 50%. Now in our case we want to have a one third split and we can do that by going the first one taking up one fraction and then we say the second one takes up two fractions.
[10:28 - 10:49] So this says is take the remaining space and divide it into three because we have three fractional units and put one of them here and give the rest to the second column or effectively one third. We'll go here and this is two thirds.
[10:50 - 11:11] And this is good we can hard code this but that's not going to be very useful. So we really want to adopt something just like we did with our spacing map property and give it some type of prop that we can say give me one third and it will automatically do what we need.
[11:12 - 11:35] So with the power and magic of copying and pays like I've been doing all along let's change it to look like this. First of all let's create a map called fractions and if we pass in one fourth then we will do one fourth at the beginning and three fourths on the end.
[11:36 - 11:50] One third one half two thirds three fourths all using the appropriate ratio. And then just because this is I found this to be very helpful let's create the concept of auto start.
[11:51 - 12:18] She says I'm going to let the first item take up the amount of room that it wants to take up and then whatever is left we'll give to the second column. And the same thing with auto end we're going to say whatever the first one column is we'll give it the remaining space and the end will let it take up the amount of room that it wants to take up.
[12:19 - 12:47] So and then right here in our grid type of columns we are doing the same thing that we're doing right here. We're going into a string interpolation we're passing in a function called props and in this case just to make it a little easier instead of doing props that getter like we did up here we are going to just pull that prop off using destructuring.
[12:48 - 13:10] And we're going to go passing the fraction and if it exists in this fraction we 'll use it otherwise we're going to default to one half which ends up being a fifty fifty split. So let's go into our split and give it a fraction equal to one third.
[13:11 - 13:24] Oops not one point three one third. And now we we've got the exact layout that we are looking for.
[13:25 - 13:37] But this getter I actually want to enforce a bigger getter. So let's actually go ahead and give it an extra large getter too.
[13:38 - 13:48] And there we go we got a little bit bigger getter in here. And there we go final code for this lesson if you like is at the end of the lesson.
[13:49 - 14:06] We've made this awesome sidebar component with a split of one third for the information and then the form takes up the other side. But this form is boring and it's ugly and really not very useful in this kind of state.
[14:07 - 14:17] So what we're going to do in the next lesson is we're going to learn about two complementary primitives to turn this into a much better form. Those primitives are the columns and column primitive.
[14:18 - 14:19] We'll see you on the next lesson.