This lesson preview is part of the Blazing Fast Next.js with React Server Components course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to Blazing Fast Next.js with React Server Components, plus 80+ \newline books, guides and courses with the \newline Pro subscription.
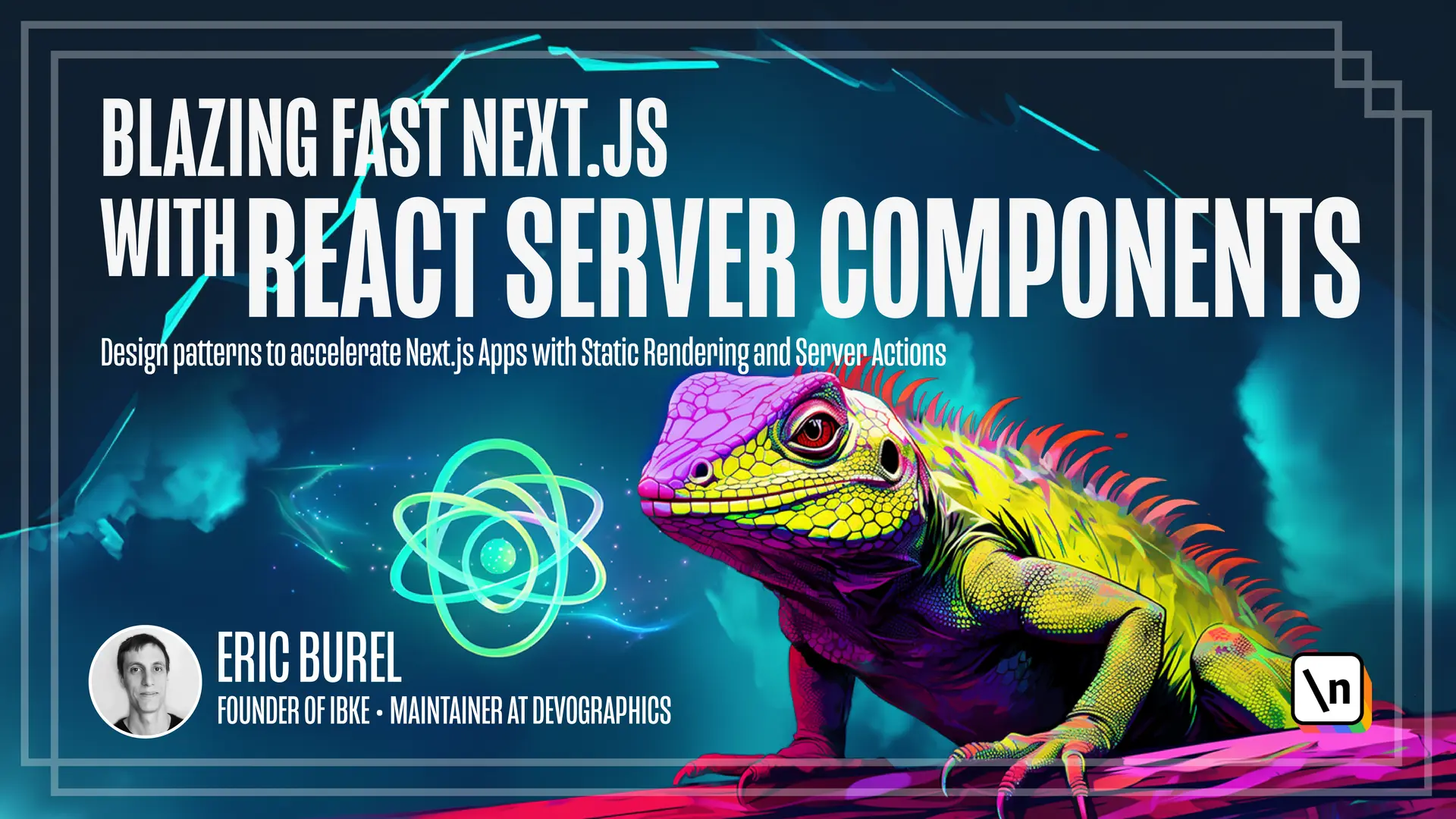
[00:00 - 00:14] The product list is a React Server Component that can get data directly from the database. Now, we want to create an administration interface that will allow us to add a new product to the product list.
[00:15 - 00:35] We often call that a mutation. This wording actually comes from GraphQL that distinguishes queries that get the data, so React Server Components in React, and mutations that can create, update, or delete some data or any other kind of server action, that we call "Server Actions" in Next.js and React.
[00:36 - 00:49] So server actions, as their name indicates, do not need any kind of client-side JavaScript to run, even though they can still be used in client-side components. But here we are going to use a server-side form with a Server Action.
[00:50 - 01:00] We are going to do everything on the server. Here we have the form, we create a new product, save the change, and we can see it on the product list.
[01:01 - 01:16] So now let's take a look at the code that implements this. So here we have the product administration area that displays two components, a product creation form and a product reset button, which is just a thing for development that allows us to reset the list of products.
[01:17 - 01:29] So let's take a look at the product creation form. Okay, if we jump to its definition, we see that it's a server component, it's not using "use client", even though it could be possible.
[01:30 - 01:43] If we enable the "use client" here, nothing happens, it just keeps working as it is. It's just that now, it will also run their client-side involving more client- side JavaScript, more computation, and thus more loading time before it's interactive.
[01:44 - 01:59] So we prefer to keep it a React Server component, which is the default and the most performant. We see that it displays the form, yeah, and it's using the "action" attribute, which is actually a default HTML attribute, it exists in any kind of form.
[02:00 - 02:16] It's not using the usual client-side "onSubmit" that I'm showing here in comments that would be way more verbose. We would need to parse the event to create a JSON, fetch to the API, so on, and then create an API route to update the form.
[02:17 - 02:29] We don't need any of that with a Server Action. Okay, we just display the form, create a button to submit the form, and then we have this Action.
[02:30 - 02:37] So let's dig what it does. So it's a server function that gets the form data as input.
[02:38 - 02:49] "FormData" is a structure from the web platform, so something that is available in JavaScript anywhere that represents the form, so I can get the different inputs of the form. I log stuff.
[02:50 - 03:04] I verify that the project is correct, because here we are server-side, so we need to validate the user inputs. Of course, we cannot trust the user, so we must check that the form has been filled correctly.
[03:05 - 03:28] Then we call the "addProduct" function, which actually comes from the database here. So if we check it out, it's just adding a product to the product list using the database helper that we have, so we can see that the "replaceProduct" is a function that updates the file where I stored the product, so it's really simulating the database.
[03:29 - 03:39] This server action is simply checking that the form data are correct, and then adding the product to the database. And we can call it like this from the server, and it just works.
[03:40 - 03:57] For this to work, Next we'll do some kind of build-time magic, so that the server-side code never leaks client-side despite the form being interactive there. You might be used to concept like RPC communication, Remote Procedure Calls.
[03:58 - 04:07] This is kind of similar. The server action is a remote procedure call that is generated on the fly by Next during compilation, basically.
[04:08 - 04:14] That's that, it's like creating an API that you don't need to care about. It just works.
[04:15 - 04:31] If you enable JavaScript, NextJS will try to be as efficient as possible and use client-side JavaScript to send the payload and update the page. And if you are naughty, it will just refresh the page, send request, and use the basic browser behavior to handle the form.
[04:32 - 04:42] Both will work the same way for the end user. This can be considered the new default way of updating, mutating data in Next JS.
[04:43 - 04:58] The security article I mentioned earlier advocates that this is actually safer than API routes, that need to be secured manually with risk of errors. API routes, route on layers in Next.js are considered a kind of fallback when the server action is not possible.
[04:59 - 05:11] Something you should not have to do too often if your NextJS app doesn't have to have a public API, you don't need an API at all. You could just use Server Actions.
[05:12 - 05:19] In theory, in practice, it might be a bit more difficult, of course. I'd like to mention here the Backend For Front-end pattern.
[05:20 - 05:50] The idea is that Next.js is a framework to craft backends for front-ends. Server Actions, React Server Components, it's really the backend for front -end paradigm, which initially has been proposed to have a kind of intermediate layer between server APIs that communicate with other servers and APIs that communicate with client -side code for interfaces because they actually have different requirements.
[05:51 - 06:05] If only the fact that APIs that must provide data to client-side code, to client-side interfaces are public. They can be accessed from the user's browser in order for the user to get some data, where server-to-server communication is different.
[06:06 - 06:14] It's private, it's happening in the safe environment or at least an environment that is secured differently. So it's really two worlds.
[06:15 - 06:25] Next really makes a point of using the server efficiently to create new front-ends. It's not really a framework to create back-ends and open APIs for other applications.
[06:26 - 06:29] The backend and the front-end are deeply integrated and that's really the idea of backend for frontend.